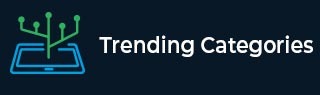
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Finding nth day of the year in leap and non-leap years in JavaScript
Problem
We are required to write a JavaScript function that takes in a number as the first argument and boolean as the second argument.
The boolean specifies a leap year (if it’s true). Based on this information our function should return the date that would fall on the nth day of the year.
Example
Following is the code −
const day = 60; const isLeap = true; const findDate = (day = 1, isLeap = false) => { if(day > 366){ return undefined; }; const months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']; const days = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]; if(isLeap){ days[1]++; }; let i = -1, count = 0; while(count < day){ i++; count += days[i]; }; const upto = days.slice(0, i).reduce((acc, val) => acc + val); const month = months[i]; const d = count - upto; return `${month}, ${d}`; }; console.log(findDate(day, isLeap));
Output
Following is the console output −
Feb, 29
Advertisements