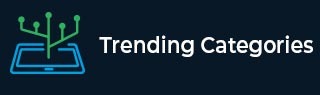
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Finding Fibonacci sequence in an array using JavaScript
Fibonacci Sequence:
A sequence X_1, X_2, ..., X_n is fibonacci if:
n >= 3
X_i + X_{i+1} = X_{i+2} for all i + 2 <= n
Problem
We are required to write a JavaScript function that takes in an array of numbers, arr, as the first and the only argument. Our function should find and return the length of the longest Fibonacci subsequence that exists in the array arr.
A subsequence is derived from another sequence arr by deleting any number of elements (including none) from arr, without changing the order of the remaining elements.
For example, if the input to the function is
Input
const arr = [1, 3, 7, 11, 14, 25, 39];
Output
const output = 5;
Output Explanation
Because the longest Fibonacci subsequence is [3, 11, 14, 25, 39]
Following is the code:
Example
const arr = [1, 3, 7, 11, 14, 25, 39]; const longestFibonacci = (arr = []) => { const map = arr.reduce((acc, num, index) => { acc[num] = index return acc }, {}) const memo = arr.map(() => arr.map(() => 0)) let max = 0 for(let i = 0; i < arr.length; i++) { for(let j = i + 1; j < arr.length; j++) { const a = arr[i] const b = arr[j] const index = map[b - a] if(index < i) { memo[i][j] = memo[index][i] + 1 } max = Math.max(max, memo[i][j]) } } return max > 0 ? max + 2 : 0 }; console.log(longestFibonacci(arr));
Output
5
Advertisements