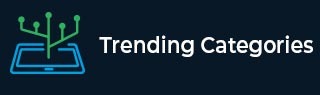
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find Values after N Operations to Remove N Characters of String βSβ With Given Constraints
What are the Specifications of Using String?
To solve a specific challenge involving the given string S. The string S contains only lowercase English letters, and certain constraints must be followed while removing characters.
The given constraints are β
There are lowercase English letters in string S.
A character can only be deleted if it appears more than once in string.
Only characters that appear consecutively can be deleted. The following steps can be used to remove characters from string S β
Find all characters that appear more than once as you iterate over string S. Find all of characterβs consecutive occurrences by iterating through string S once again for every character.
Remove the first N occurrences of character if number of consecutive occurrences is higher than or equal to iteration number.
Continue performing steps 2 and 3 until all iterations are finished.
Finally, by returning final string S, value of string after N operations to remove N characters can be discovered.
Syntax
This topic is a coding problem which involves manipulating a given string by performing a certain number of operations on it. In each operation, the most frequent character in string is removed, and frequency of each remaining character is updated. After performing N operations, the final value of string is calculated by squaring the frequencies of each remaining character and summing them up. The goal of the problem is to write a program that takes a string and number N as input and outputs final value of string after performing N operations according to given constraints.
Below is the syntax for function that finds value after N operations to remove N characters of string S with given constraints β
int findvalueafterNoperations(int n, string s) { int len = s.length(); int freq[26] = {0}; for (int i = 0; i < len; i++) { freq[s[i] - 'a']++; } sort(freq, freq + 26, greater<int>()); for (int i = 0; i < n; i++) { freq[0]--; sort(freq, freq + 26, greater<int>()); } int value = 0; for (int i = 0; i < 26; i++) { value += freq[i] * freq[i]; } return value; }
This function takes in two arguments β
n β integer representing number of operations to perform.
s β string representing the input string.
The function first calculates frequency of each character in input string using an array. Then sorts this frequency array in descending order and performs N operations, where it decrements frequency of most frequent character in each operation and sorts the frequency array again.
Finally, function calculates value of string by summing squares of frequencies of each character in sorted frequency array and returns it as integer.
Algorithm
After N processes of character removal, the algorithm calculates the value of string under the following limitations. The input consists of the number N and a string S.
Step 1 β Using an array, determine the frequency of each character in the input string.
Step 2 β Descending order sort this frequency array.
Step 3 β Carry out N operations, lowering the frequency of the character that appears the most frequently in the frequency array with each operation.
Step 4 β Rearrange the frequency array.
Step 5 β Add the squares of the frequencies of each character in the sorted frequency array to determine the string's value.
Step 6 β After N operations, the string's value is the sum of its squares.
This technique works because problem calls for the removal of N characters from the input string S, which is like carrying out N operations in which most frequent character in the string is removed once for each operation. We cannot truly remove characters from string due to limits of the task, so we must simulate this action by decreasing frequency of most common character in the frequency array throughout each operation.
Approaches to Follow
Approach 1
A sample string S and various operations N are initialized using the code. The initial character that is greater than its next character is then removed after looping through each operation. The final character is eliminated if none are removed. Following conclusion of all actions, it prints the string's final value.
Here, the code assumes that N is either shorter or equal to length of string S. If N is longer than S the code will not function as intended.
Example-1
#include <iostream> #include <string> using namespace std; int main(){ string S = "abcdefg"; int N = 3; for (int l = 1; l <= N; l++) { int p=0; while(p<S.length()- 1) { if(S[p]>S[p+1]) { S.erase(p, 1); break; } p++; } if(p==S.length()- 1) { S.erase(p, 1); } } cout<< S << endl; return 0 ; }
Output
a b c d
Approach-2
In this code, frequency of each character in input string is first determined using an array. We next execute N operations, decrementing frequency of most frequent character in each operation, and sort frequency array once again. Next, we sort this frequency array in decreasing order.
The value of string is finally determined by adding squares of frequencies of each character in sorted frequency array.
Example-2
#include <iostream> #include <algorithm> #include <string> using namespace std; int main(){ // Given values int n = 3; string s = "abcabc"; int len = s.length(); int freq[26] = {0}; for (int i = 0; i < len; i++) { freq[s[i] - 'a']++; } sort(freq, freq + 26, greater<int>()); for (int i = 0; i < n; i++) { freq[0]--; sort(freq, freq + 26, greater<int>()); } int value = 0; for (int i = 0; i < 26; i++) { value += freq[i] * freq[i]; } cout << "Value of string after " << n << " operations: " << value << endl; return 0; }
Output
Value of string after 3 operations: 3
Conclusion
In conclusion, we can use straight forward technique to get values after N operations to eliminate N characters from string "S" with above restrictions. To begin, let's initialize frequency array to keep track of how many characters there are in string. Once we have eliminated N characters, we can repeat the process of removing character with greatest count from frequency array. This procedure can be repeated a total of N times.
With the help of this approach, we may quickly determine values for the string "S" after N operations that include eliminating N characters. Due to the sorting phase in the method, this solution has a time complexity of O (N logN), which is acceptable for most real applications.