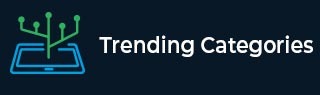
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find two distinct prime numbers with given product in C++
In this tutorial, we will be discussing a program to find two distinct prime numbers with given product.
For this we will be provided with an integer value. Our task is to find the two prime integer values such that their product is equal to the given value.
Example
#include <bits/stdc++.h> using namespace std; //generating prime numbers less than N. void findingPrimeNumbers(int n, bool calcPrime[]) { calcPrime[0] = calcPrime[1] = false; for (int i = 2; i <= n; i++) calcPrime[i] = true; for (int p = 2; p * p <= n; p++) { if (calcPrime[p] == true) { for (int i = p * 2; i <= n; i += p) calcPrime[i] = false; } } } //printing the valid prime pair void calcPairPrime(int n) { int flag = 0; bool calcPrime[n + 1]; findingPrimeNumbers(n, calcPrime); for (int i = 2; i < n; i++) { int x = n / i; if (calcPrime[i] && calcPrime[x] and x != i and x * i == n) { cout << i << " " << x; flag = 1; return; } } if (!flag) cout << "No prime pair exist"; } int main() { int n = 24; calcPairPrime(n); return 0; }
Output
No prime pair exist
Advertisements