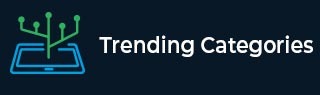
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the sum of maximum difference possible from all subset of a given array in Python
Suppose we have an array A of n values (elements may not be distinct). We have to find the sum of maximum difference possible from all subsets of given array. Now consider max(s) denotes the maximum value in any subset, and min(s) denotes the minimum value in the set. We have to find the sum of max(s)-min(s) for all possible subsets.
So, if the input is like A = [1, 3, 4], then the output will be 9.
To solve this, we will follow these steps −
n := size of A
sort the list A
sum_min := 0, sum_max := 0
for i in range 0 to n, do
sum_max := 2 * sum_max + A[n-1-i]
sum_max := sum_max mod N
sum_min := 2 * sum_min + A[i]
sum_min := sum_min mod N
return(sum_max - sum_min + N) mod N
Example
Let us see the following implementation to get better understanding −
N = 1000000007 def get_max_min_diff(A): n = len(A) A.sort() sum_min = 0 sum_max = 0 for i in range(0,n): sum_max = 2 * sum_max + A[n-1-i] sum_max %= N sum_min = 2 * sum_min + A[i] sum_min %= N return (sum_max - sum_min + N) % N A = [1, 3, 4] print(get_max_min_diff(A))
Input
[1, 3, 4]
Output
9
Advertisements