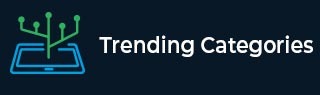
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the smallest number formed by inserting a given digit
Inserting a number in the given number means adding a new given digit in the given number either at the front, end, or in between the numbers. We have given a number and a digit and have to add the digit in the number in a way such that the resultant new number will be minimum as possible. We will convert the number into a string to make the work of insertion easy. Also, the given number can be negative also so we have to consider this case.
Sample Examples
Input1
Given number: 124 Given digit: 3 Output: 1234
Explanation − We have four places where we can add the given number and the results can be 3124, 1324, 1234, 1243. Among all four the second last is the minimum.
Input2
Given number: -124 Given digit: 3 Output: -3124
Explanation − We have four places where we can add the given number and the results can be -3124, -1324, -1234, -1243. Among all four the first one is the minimum.
Naive Approach
We have seen the examples now let us move on to see the steps we will perform to solve the problem −
First, we will check whether the current number is a positive or negative number.
If the current number is negative then we will mark it a variable as negative and make the current number as positive.
After that we will convert the current number to a string and call the function basis of the current number as negative or positive.
In the functions we will try to fit the digit at every place and will check if the current whether the current number is smaller or greater on the basis of the positive or negative number.
If the current number is positive, we will try to find the number that which is smallest and will return that.
Else, we will find the greatest number and return it by multiplying by -1.
Example
#include <bits/stdc++.h> using namespace std; int findMin(string str, int d){ string ans = str + to_string(d); // variable to store the answer // traversing over the string for(int i=0; i<= str.size(); i++){ ans = min(ans, str.substr(0,i) + to_string(d) + str.substr(i)); } return stoi(ans); } int findMax(string str, int d){ string ans = str + to_string(d); // variable to store the answer // traversing over the string for(int i=0; i<= str.size(); i++){ ans = max(ans, str.substr(0,i) + to_string(d) + str.substr(i)); } return stoi(ans); } int minimumNumber(int n, int d){ // checking for the negative number int isNeg = 1; if(n < 0){ n *= -1; isNeg = -1; } // converting the current number to string string str = to_string(n); if(isNeg == 1){ return findMin(str,d); } else{ return -1*findMax(str,d); } } int main(){ int n = -124; // given number int d = 3; // given digit // calling to the function n = minimumNumber(n, d); cout<<"The minimum number after adding the new digit is "<<n<<endl; return 0; }
Output
The minimum number after adding the new digit is -3124
Time and Space Complexity
The Time complexity of the above code is O(N*N) where N is the number of digits in the given number.
The space complexity of the above code is O(N) where N is the number of digits in the given number.
Efficient Approach
In the previous approach, we were going over the digit and checking for each digit but the efficient method is to just find the first digit which is greater as compared to the given digit for the positive number and add it and return itself. For the negative number find the find digit in the number which is smaller as compared to it and add it and return that.
Let us see the code −
Example
#include <bits/stdc++.h> using namespace std; int findMin(string str, int d){ // traversing over the string for(int i=0; i<= str.size(); i++){ if(str[i]-'0' > d){ return stoi(str.substr(0,i) + to_string(d) + str.substr(i)); } } return stoi(str + to_string(d)); } int findMax(string str, int d){ // traversing over the string for(int i=0; i<= str.size(); i++){ if(str[i]-'0' < d){ return stoi(str.substr(0,i) + to_string(d) + str.substr(i)); } } return stoi(str + to_string(d)); } int minimumNumber(int n, int d){ // checking for the negative number int isNeg = 1; if(n < 0){ n *= -1; isNeg = -1; } // converting the current number to string string str = to_string(n); if(isNeg == 1){ return findMin(str,d); } else{ return -1*findMax(str,d); } } int main(){ int n = 124; // given number int d = 3; // given digit // calling to the function n = minimumNumber(n, d); cout<<"The minimum number after adding the new digit is "<<n<<endl; return 0; }
Output
The minimum number after adding the new digit is 1234
Time and Space Complexity
The Time complexity of the above code is O(N) where N is the number of digits in the given number.
The space complexity of the above code is O(N) where N is the number of digits in the given number.
Conclusion
In this tutorial, we have implemented an approach to inserting a number in the given number means adding a new given digit in the given number either at the front, end, or in between the numbers. We have seen two approaches one with the time complexity of the O(N*N) and another with the O(N). Space complexity of both the approaches is O(N).