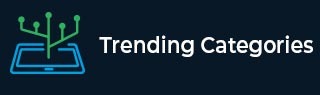
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the player who rearranges the characters to get a palindrome string first in Python
Suppose we have a string S with lowercase letters, now two players are playing the game. The rules are as follows −
The player wins the game, if, at any move, a player can shuffle the characters of the string to get a palindrome string.
The player cannot win when he/she has to remove any character from the string.
We have to keep in mind that both players play the game optimally and player1 starts the game. We have to find the winner of the game.
So, if the input is like "pqpppq", then the output will be Player1 as player-1 in the first step arranges the characters to get “ppqqpp” and wins the game.
To solve this, we will follow these steps −
l := size of sequence
freq := make a list of size 26 and filled with 0
for i in range 0 to l, increase by 1, do
increase freq of sequence[i] by 1
count := 0
for i in range 0 to 25, do
if freq[i] mod 2 is not 0, then
count := count + 1
if count is 0 or count is odd, then
return 1
otherwise,
return 2
Example
Let us see the following implementation to get better understanding −
def who_is_the_winner(sequence): l = len(sequence) freq = [0 for i in range(26)] for i in range(0, l, 1): freq[ord(sequence[i]) - ord('a')] += 1 count = 0 for i in range(26): if (freq[i] % 2 != 0): count += 1 if (count == 0 or count & 1 == 1): return 1 else: return 2 sequence = "pqpppq" print("Player:", who_is_the_winner(sequence) )
Input
"pqpppq"
Output
Player: 1