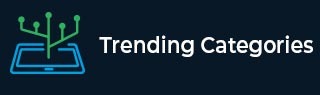
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the one missing number in range using C++
In this problem, we are given an arr[] of size n. Our task is to find the one missing number in range.
The array consists of all values ranging from smallest value to (smallest + n). One element of the range is missing from the array. And we need to find this missing value.
Let’s take an example to understand the problem,
Input
arr[] = {4, 8, 5, 7}
Output
6
Solution Approach
A simple solution to the problem is by searching the missing element by sorting the array and then finding the first element of range starting from minimum value which is not present in the array but present in the range.
This solution is a naive approach and will solve the problem is O(n log n) time complexity.
Another approach to solving the problem in less time is using XOR of the values of the array and the range. We will find the XOR of all values in the range and also find the XOR of all values of the array. The XOR of both these values will be our missing value.
Example
Program to illustrate the working of our solution
#include <bits/stdc++.h> using namespace std; int findMissingNumArr(int arr[], int n){ int arrMin = *min_element(arr, arr+n); int numXor = 0; int rangeXor = arrMin; for (int i = 0; i < n; i++) { numXor ^= arr[i]; arrMin++; rangeXor ^= arrMin; } return numXor ^ rangeXor; } int main(){ int arr[] = { 5, 7, 4, 8, 9}; int n = sizeof(arr)/sizeof(arr[0]); cout<<"The missing value in the array is "<<findMissingNumArr(arr, n); return 0; }
Output
The missing value in the array is 6