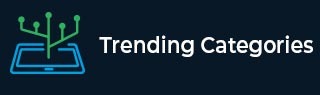
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the Number of Triangles Formed from a Set of Points on Three Lines using C++
We are given several points present in 3 lines now; we are required to find how many triangles these points can form, for example
Input: m = 3, n = 4, k = 5 Output: 205 Input: m = 2, n = 2, k = 1 Output: 10
We will apply some combinatorics to this question and make up some formulas to solve this problem.
Approach to find The Solution
In this approach, we will devise a formula by applying combinatorics to the current situations, and this formula will give us our results.
C++ Code for the Above Approach
Here is the C++ syntax which we can use as an input to solve the given problem −
Example
#include <bits/stdc++.h> #define MOD 1000000007 using namespace std; long long fact(long long n) { if(n <= 1) return 1; return ((n % MOD) * (fact(n-1) % MOD)) % MOD; } long long comb(int n, int r) { return (((fact(n)) % MOD) / ((fact(r) % MOD) * (fact(n-r) % MOD)) % MOD); } int main() { int n = 3; int m = 4; int r = 5; long long linen = comb(n, 3); // the combination of n with 3. long long linem = comb(m, 3); // the combination of m with 3. long long liner = comb(r, 3); //the combination of r with 3. long long answer = comb(n + m + r, 3); // all possible comb of n, m , r with 3. answer -= (linen + linem + liner); cout << answer << "\n"; return 0; }
Output
205
Explanation of the above code
In this approach, we find all the possible combinations of n+m+r with three, i.e., comb(n+m+r, 3). Now, as you know, the conditions for 3 points to be a triangle is that they shouldn't be collinear, so we find all the possible collinear points that are the sum of the combination of n, m, r with three, and when we subtract this sum with the variety of n+m+r with three we get the answer, and we print it.
Conclusion
This article discussed how many triangles can be formed from a set of points on three lines by applying some combinatorics. We also learned the C++ program for this problem and the complete approach (Normal) by which we solved this problem. We can write the same program in other languages such as C, java, python, and other languages. We hope you find this article helpful.