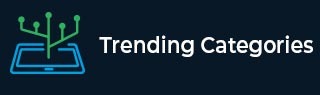
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the Number of Subarrays whose Minimum and Maximum are Same using C++
In this article, we will solve the problem of finding the number of subarrays whose maximum and minimum elements are the same using C++. Here is the example for the problem −
Input : array = { 2, 3, 6, 6, 2, 4, 4, 4 } Output : 12 Explanation : {2}, {3}, {6}, {6}, {2}, {4}, {4}, {4}, {6,6}, {4,4}, {4,4} and { 4,4,4 } are the subarrays which can be formed with maximum and minimum element same. Input : array = { 3,3,1,5,1,2,2 } Output : 9 Explanation : {3}, {3}, {1}, {5}, {1}, {2}, {2}, {3,3} and {2,2} are the subarrays which can be formed with minimum and maximum are the same.
Approach to Find Solution
Looking at examples, we can say the minimum number of subarrays can be formed with the same minimum and maximum elements equal to the size of the array. The number of subarrays can be more if there are the same consecutive numbers.
So we can apply an approach of going through every element and check whether its consecutive numbers are the same or not and increment count if consecutive numbers are the same, and break the inner loop if a different number is found.
The result variable increases the result variable every time the inner loop ends or breaks and finally shows the result from the result variable.
Example
#include <bits/stdc++.h> using namespace std; int main(){ int a[ ] = { 2, 4, 5, 3, 3, 3 }; int n = sizeof(a) / sizeof(a[0]); int result = n, count =0; for (int i = 0; i < n; i++) { for (int j = i+1; j < n; j++) { if(a[i]==a[j]) count++; else break; } result+=count; count =0; } cout << "Number of subarrays having minimum and maximum elements same:" << result; return 0; }
Output
Number of subarrays having minimum and maximum elements same: 9 Time complexity = O(n2).
Explanation of the Above code
In this code, we are taking variable n to store the size of the array, result = n, because minimum n subarrays can be formed and counted to keep count of the same numbers.
The outer loop is used to process every element in an array. The inner loop is used to find how many consecutive numbers are the same after the index element and incrementing the result variable with the count variable every time the inner loop ends. Finally showing the output stored in the result variable.
Efficient approach
In this approach, we are going through every element, and for every element, we are searching how many consecutive same numbers are there. For each same number found, we are incrementing count variable, and when different numbers are found then with the help of count, we are finding how many subarrays can be formed using the formula "n = n*(n+1)/2" and incrementing the result variable with the answer of this formula.
Example
#include <bits/stdc++.h> using namespace std; int main(){ int a[] = { 2, 4, 5, 3, 3, 3 }; int n = sizeof(a) / sizeof(a[0]); int result = 0; int count =1,temp=a[0]; for (int i = 1; i < n; i++) { if (temp==a[i]){ count++; } else{ temp=a[i]; result = result + (count*(count+1)/2); count=1; } } result = result + (count*(count+1)/2); cout << "Number of subarrays having minimum and maximum elements same:" << result; return 0; }
Output
Number of subarrays having minimum and maximum elements same: 9 Time complexity : O(n)
Explanation of the Above code
In this code, we store the 0th index of the array in the temp variable and start the loop with index 1. We check whether the temp variable is equal to the element at the current index and incrementing count by 1 for the same number found. If the temp variable is not equal to the index element, then we find the combinations of subarrays that can be made from the count of the same numbers and storing the result in the result variable. We change the temp value to the current index resetting count to 1. Finally, we are showing the answer stored in the result variable.
Conclusion
In this article, we solve a problem to find the Number of subarrays whose minimum and maximum elements are the same. We also learned the C++ program for this problem and the complete approach ( Normal and efficient) by which we solved this problem. We can write the same program in other languages such as C, java, python, and other languages. Hope you find this article helpful.