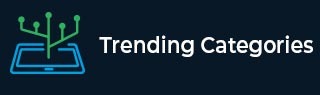
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the number of stair steps using C++
In this problem, we are given a number N denoting the number of bricks provided to create the stair. Our task is to find the number of stair steps.
Using the given bricks, we need to create a stair step. Each step takes one more brick that the last step. And the first step is two bricks high. We need to find the number of such steps that can be made from bricks.
Let’s take an example to understand the problem,
Input
N = 40
Output
3
Explanation
Step = 1 ; bricks required = 2; total bricks used = 2 ; bricks remaining = 38
Step = 2 ; bricks required = 3; total bricks used = 5 ; bricks remaining = 35
Step = 3 ; bricks required = 4; total bricks used = 9 ; bricks remaining = 31
Step = 4 ; bricks required = 5; total bricks used = 14 ; bricks remaining = 26
Step = 5 ; bricks required = 6; total bricks used = 20 ; bricks remaining = 20
Step = 6 ; bricks required = 7; total bricks used = 27 ; bricks remaining = 13
Step = 7 ; bricks required = 8; total bricks used = 35 ; bricks remaining = 5
No further steps can be created as 8th steps require 9 bricks and only 5 are present.
Solution Approach
A simple solution to the problem is by using a loop, starting from 2 till the number of bricks used surpasses N and then return the last step’s count.
This solution is good but the time complexity is of the order N. And a better approach can be made to find the solution using the sum formula and binary search.
We have X which is the total count of bricks to be used which is always created than (n*(n+1)) /2 as we have the sum of all bricks required. In this case, we have a solution from either of two values which is found from mid and mid - 1.
Example
Program to illustrate the working of our solution
#include <iostream> using namespace std; int findStairCount(int T){ int low = 1; int high = T/2; while (low <= high) { int mid = (low + high) / 2; if ((mid * (mid + 1)) == T) return mid; if (mid > 0 && (mid * (mid + 1)) > T && (mid * (mid - 1)) <= T) return mid - 1; if ((mid * (mid + 1)) > T) high = mid - 1; else low = mid + 1; } return -1; } int main(){ int N = 60; int stepCount = findStairCount(2*N); if (stepCount != -1) stepCount--; cout<<"The number of stair steps that can be made is "<<stepCount; return 0; }
Output
The number of stair steps that can be made is 9