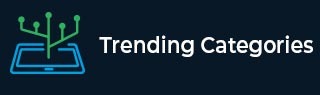
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the number of spectators standing in the stadium at time t in Python
There are n number of spectators in the stadium, and they are labeled from 1 to n. Now follow these cases −
At time t1, the first spectator stands.
At time t2, the second spectator stands.
…
At time tk, the k-th spectator stands.
At time tk + 1, the (k + 1)-th spectator stands and the first spectator sits.
At time tk + 2, the (k + 2)-th spectator stands and the second spectator sits.
…
At time tn, the n-th spectator stands and the (n – k)-th spectator sits.
At time tn + 1, the (n + 1 – k)-th spectator sits.
…
At time tn + k, the n-th spectator sits.
So, if the input is like n = 11, k = 6, t = 4, then the output will be 4 as at time t1, 1st spectator stands, then at time t2, 2nd spectator stands after that at time t3, 3rd spectator stands, and finally at time t4, 4th spectator stands. In this way the result is 4 as there are 4 spectators standing in total.
To solve this, we will follow these steps −
if t <= k, then
return t
otherwise when t <= n, then
return k
otherwise,
res := t - n
res := k - res
return res
Example
Let us see the following implementation to get better understanding −
def how_many_stand(n, k, t) : if (t <= k) : return t elif (t <= n) : return k else : res = t - n res = k - res return res n = 11 k = 6 t = 4 print(how_many_stand(n, k, t))
Input
11, 6, 4
Output
4