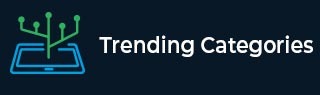
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the number of islands Using DFS in C++
In this problem, we are given a 2D binary matrix. Our task is to Find the number of islands Using DFS.
Island is a ground of 1 or more connected 1’s in the matrix.
Let’s take an example to understand the problem,
Input : bin[][] = {{ 1 0 0 0} {0 1 0 1} {0 0 0 0} {0 0 1 0}} Output : 3Explanation
Islands are −bin00 - bin11
bin13
bin32
Solution Approach
To solve the problem using DFS, we will use the DFS technique for exploring all the neighbours (maximum possible 8 of a number in the matrix) and check for 1’s. If we encounter 1 value which is unvisited, then we will consider it. We will keep a check on the values that are visited to avoid repeated visits. Doing so, we can count the number of islands present in the matrix.
Example
#include <bits/stdc++.h> using namespace std; #define ROW 5 #define COL 5 int canVisit(int bin[][COL], int row, int col, bool visited[][COL]){ return (row >= 0) && (row < ROW) && (col >= 0) && (col < COL) && (bin[row][col] && !visited[row][col]); } void DFS(int bin[][COL], int row, int col, bool visited[][COL]){ static int getNeighbourRow[] = { -1, -1, -1, 0, 0, 1, 1, 1 }; static int getNeighbourCol[] = { -1, 0, 1, -1, 1, -1, 0, 1 }; visited[row][col] = true; for (int k = 0; k < 8; ++k) if (canVisit(bin, row + getNeighbourRow[k], col + getNeighbourCol[k], visited)) DFS(bin, row + getNeighbourRow[k], col + getNeighbourCol[k], visited); } int findIslandCount(int bin[][COL]){ bool visited[ROW][COL]; memset(visited, 0, sizeof(visited)); int islandCount = 0; for (int i = 0; i < ROW; ++i) for (int j = 0; j < COL; ++j) if (bin[i][j] && !visited[i][j]) { DFS(bin, i, j, visited); islandCount++; } return islandCount; } int main(){ int bin[][COL] = {{1, 0, 0, 0}, {0, 1, 0, 1}, {0, 0, 0, 0}, {0, 0, 1, 0}}; cout<<"The number of islands present in the matrix is "<<findIslandCount(bin); return 0; }
Output
The number of islands present in the matrix is 4
Advertisements