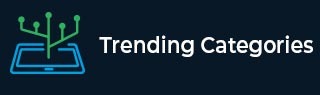
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the number of consecutive zero at the end after multiplying n numbers in Python
Suppose we have an array with n numbers, we have to return the number of consecutive zero’s at the end after multiplying all the n numbers.
So, if the input is like [200, 20, 5, 30, 40, 14], then the output will be 6 as 200 * 20 * 5 * 30 * 40 * 14 = 336000000, there are six 0s at the end.
To solve this, we will follow these steps −
Define a function count_fact_two() . This will take n
count := 0
while n mod 2 is 0, do
count := count + 1
n := n / 2 (only the quotient as integer)
return count
Define a function count_fact_five() . This will take n
count := 0
while n mod 5 is 0, do
count := count + 1
n := n / 5 (only the quotient as integer)
return count
From the main method, do the following −
n := size of A
twos := 0, fives := 0
for i in range 0 to n, do
twos := twos + count_fact_two(A[i])
fives := fives + count_fact_five(A[i])
if twos − fives, then
return twos
otherwise,
return fives
Example
Let us see the following implementation to get better understanding −
def count_fact_two( n ): count = 0 while n % 2 == 0: count+=1 n = n // 2 return count def count_fact_five( n ): count = 0 while n % 5 == 0: count += 1 n = n // 5 return count def get_consecutive_zeros(A): n = len(A) twos = 0 fives = 0 for i in range(n): twos += count_fact_two(A[i]) fives += count_fact_five(A[i]) if twos < fives: return twos else: return fives A = [200, 20, 5, 30, 40, 14] print(get_consecutive_zeros(A))
Input
[200, 20, 5, 30, 40, 14]
Output
6