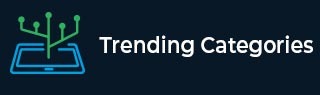
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the Number of 1 Bits in a large Binary Number in C++
Given a 32-bit Unsigned Binary Number, the task is to count the set bits, i.e., '1's present in it.
For Example
Input:
N = 00000000000000100111
Output:
4
Explanation: Total set bits present in the given unsigned number is 4, thus we will return the output as '4'.
Approach to Solve this Problem
We have given an unsigned 32-bit binary number. The task is to count how many '1's present in it.
To count the number of '1's present in the given binary number, we can use the inbuilt STL function '__builin_popcount(n)' which takes a binary number as the input parameter.
- Take a binary number N as the input.
- A function count1Bit(uint32_t n) takes a 32-bit binary number as the input and returns the count of '1' present in the binary number.
- The inbuilt function __builtin_popcount(n) takes the input of 'n' as a parameter and returns the count.
Example
#include<bits/stdc++.h> using namespace std; int count1bits(uint32_t n) { return bitset < 32 > (n).count(); } int main() { uint32_t N = 0000000010100000011; cout << count1bits(N) << endl; return 0; }
Running the above code will generate the output as,
Output
4
In the given number, there are 4 set bits or '1s' present in it. So, the output is '4'.
Advertisements