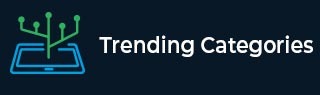
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the missing value from the given equation a + b = c in Python
Suppose we have one equation in this form: a + b = c, now any one of the terms of a, b or c is missing. We have to find the missing one.
So, if the input is like ? + 4 = 9, then the output will be 5
To solve this, we will follow these steps −
delete all blank spaces from the string and change (+ and = to comma ',')
elements := a list of elements by splitting the string separated by comma
idx := 0
for i in range 0 to size of elements, do
if elements[i] is not numeric, then
idx := i
come out from the loop
if last element is missing, then
return first element + second element
otherwise when second element is missing, then
return last element - first element
otherwise when first element is missing, then
return last element - second element
Example
Let us see the following implementation to get better understanding −
def find_missing(string): string = string.strip().replace(' ', '') string = string.replace('=',',') string = string.replace('+',',') elements = string.split(',') idx = 0 for i in range(len(elements)): if not elements[i].isnumeric(): idx = i break if idx == 2: return int(elements[0]) + int(elements[1]) elif idx == 1: return int(elements[2]) - int(elements[0]) elif idx == 0: return int(elements[2]) - int(elements[1]) print(find_missing('6 + 8 = ?')) print(find_missing('? + 8 = 20')) print(find_missing('5 + ? = 15'))
Input
'6 + 8 = ?' '? + 8 = 20' '5 + ? = 15'
Output
14 12 10