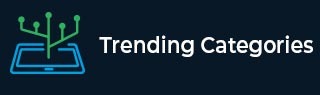
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the maximum possible value of the minimum value of modified array in C++
In this problem, we are given an array arr[] of size n and a number S. Our task is to Find the maximum possible value of the minimum value of the modified array.
Here, are the rules to modify the array,
The difference between the sum of the array elements before and after modification should be S.
Negative values in the modified array are not allowed.
If the modified array, the minimum values of the array need to be maximized.
The modification of the array can be done by increasing or decreasing any element of the array.
Using these constraints, we need to find the new array and return the maximised value for the smallest element of the array.
Let’s take an example to understand the problem,
Input : arr[] = {4, 5, 6} S = 2 Output : 4
Explanation
the modified array is {4, 5, 5}
Solution Approach
We need to maximise the smallest value of the modified array. We will do this using the binary search of the optimum value for the smallest value which is between 0 (smallest possible) and arrmin (largest possible). We will check the difference for the smallest possible value.
Some special condition,
If S is greater than array sum, no solution is possible.
If S is equal to array sum, 0 will be the value of the minimum element.
Example
Program to illustrate the working of our solution
#include <iostream> using namespace std; int findmaximisedMin(int a[], int n, int S){ int minVal = a[0]; int arrSum = a[0]; for (int i = 1; i < n; i++) { arrSum += a[i]; minVal = min(a[i], minVal); } if (arrSum < S) return -1; if (arrSum == S) return 0; int s = 0; int e = minVal; int ans; while (s <= e) { int mid = (s + e) / 2; if (arrSum - (mid * n) >= S) { ans = mid; s = mid + 1; } else e = mid - 1; } return ans; } int main(){ int a[] = { 4, 5, 6 }; int S = 2; int n = sizeof(a) / sizeof(a[0]); cout<<"The maximum value of minimum element of the modified array is "<<findmaximisedMin(a, n, S); return 0; }
Output
The maximum value of minimum element of the modified array is 4