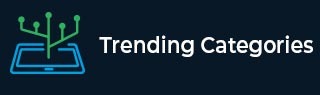
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the Longest Substring Containing Vowels in Even Counts in C++
Suppose we have the string s, we have to find the size of the longest substring containing each vowel an even number of times. That is, 'a', 'e', 'i', 'o', and 'u' must appear an even number of times. So if the string is like “helloworld”, then the output will be 8.
To solve this, we will follow these steps −
ret := 0, define two maps m and cnt, set m[“00000”] := -1
store vowels into vowels array
for i in range 0 to size of s
x := s[i], and ok := false
increase cnt[x] by 1, set temp := empty string
for k in range 0 to 4: temp := temp + ‘0’ + cnt[vowels[k]] mod 2
if m has temp, then ret := max of ret and i – m[temp], otherwise m[temp] := i
return ret
Example (C++)
Let us see the following implementation to get better understanding −
#include <bits/stdc++.h> using namespace std; class Solution { public: int findTheLongestSubstring(string s) { int ret = 0; map <string, int> m; map <char, int> cnt; m["00000"] = -1; char vowels[5] = {'a', 'e', 'i', 'o', 'u'}; for(int i = 0; i < s.size(); i++){ char x = s[i]; bool ok = false; cnt[x]++; string temp = ""; for(int k = 0; k < 5; k++){ temp+= ('0' + (cnt[vowels[k]] % 2)); } if(m.count(temp)){ ret = max(ret, i - m[temp]); } else{ m[temp] = i; } } return ret; } }; main(){ Solution ob; cout << (ob.findTheLongestSubstring("helloworld")); }
Input
“helloworld”
Output
8
Advertisements