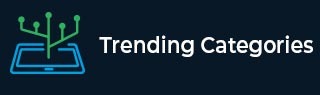
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the GCD of an array made up of numeric strings
In this article, we'll delve into an intriguing problem related to arrays and string manipulation. The problem we're examining today is "Find the GCD (Greatest Common Divisor) of an array made up of numeric strings". This problem is a great way to hone your skills in string manipulation, arrays, and number theory.
Problem Statement
Given an array of strings where each string represents a positive integer, our task is to find the Greatest Common Divisor (GCD) of all these integers.
Approach
We'll convert each string to an integer and calculate the GCD of all these integers. To calculate the GCD, we can use Euclid's algorithm, which is an efficient way to find the GCD of two numbers.
Example
Here're the programs that solves the problem −
#include <stdio.h> #include <stdlib.h> // Function to calculate the greatest common divisor (GCD) using Euclidean algorithm int gcd(int a, int b) { if (b == 0) { return a; } return gcd(b, a % b); } // Function to find the GCD of an array of integers int findGCD(int arr[], int size) { int result = arr[0]; // Loop through the array and update the GCD with each element for (int i = 1; i < size; i++) { result = gcd(result, arr[i]); } return result; } int main() { int arr[] = {42, 84, 126, 168}; int size = sizeof(arr) / sizeof(arr[0]); int result = findGCD(arr, size); printf("The GCD of the array is: %d\n", result); return 0; }
Output
The GCD of the array is: 42
#include <iostream> #include <vector> #include <algorithm> #include <string> using namespace std; int gcd(int a, int b) { if (b == 0) { return a; } return gcd(b, a % b); } int findGCD(vector<string>& arr) { int result = stoi(arr[0]); for(int i = 1; i < arr.size(); i++) { result = gcd(result, stoi(arr[i])); } return result; } int main() { vector<string> arr = {"42", "84", "126", "168"}; int result = findGCD(arr); cout << "The GCD of the array is: " << result << endl; return 0; }
Output
The GCD of the array is: 42
import java.util.Arrays; public class GCDProgram { // Function to calculate the greatest common divisor (GCD) using Euclidean algorithm public static int gcd(int a, int b) { if (b == 0) { return a; } return gcd(b, a % b); } // Function to find the GCD of an array of integers public static int findGCD(int[] arr) { int result = arr[0]; // Loop through the array and update the GCD with each element for (int i = 1; i < arr.length; i++) { result = gcd(result, arr[i]); } return result; } public static void main(String[] args) { int[] arr = {42, 84, 126, 168}; int result = findGCD(arr); System.out.println("The GCD of the array is: " + result); } }
Output
The GCD of the array is: 42
# Function to calculate the greatest common divisor (GCD) using Euclidean algorithm def gcd(a, b): if b == 0: return a return gcd(b, a % b) # Function to find the GCD of a list of integers def find_gcd(arr): result = int(arr[0]) # Loop through the list and update the GCD with each element for i in range(1, len(arr)): result = gcd(result, int(arr[i])) return result if __name__ == "__main__": arr = ["42", "84", "126", "168"] result = find_gcd(arr) print("The GCD of the array is:", result)
Output
The GCD of the array is: 42
Explanation with a Test Case
Let's take the array of strings {"42", "84", "126", "168"}.
When we pass this array to the findGCD function, it first converts the first string to an integer and stores it in the variable result.
Then it iterates over the rest of the array, converting each string to an integer and updating result to be the GCD of result and the current integer.
For the given array, the GCD of all the integers is 42. So, the function will return 42.
Conclusion
This problem demonstrates how we can manipulate strings and use number theory to solve complex problems in C++. It's an excellent problem to practice your C++ coding skills and to understand Euclid's algorithm for finding the GCD of two numbers.