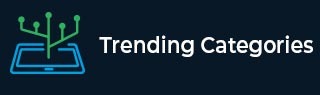
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the first repeating element in an array of integers C++
In this problem, we are an array arr of n integer values. Our task is to find the first repeating element in an array of integers.
We need to find the first integer value from the array which occurred more than once in the array.
Let's take an example to understand the problem,
Input : arr[] = {4, 1, 8, 9, 7, 2, 1, 6, 4} Output : 4
Explanation −
Integers with more than one occurrence are 4 and 1. 4's first occurrence is smaller than 1. Hence the answer is 4
Solution Approach
A simple solution to the problem is using nested loops. We will use two loops, outer one to iterate each integer value of the array and the inner one to check if there is another integer value present in the array with the same value. If yes, return the value. This method is good but in case of no solution, it will have O(N2) complexity.
Solution Approach
Another approach which can solve the problem better is by using hashing. We will traverse the array from the last index and then update the minimum index for the element found at the index which is visited.
Example
Program to illustrate the working of our solution
#include<bits/stdc++.h> using namespace std; int findRepeatingElementArray(int arr[], int n){ int minRetIndex = -1; set<int> visitedElements; for (int i = n - 1; i >= 0; i--){ if (visitedElements.find(arr[i]) != visitedElements.end()) minRetIndex = i; else visitedElements.insert(arr[i]); } if (minRetIndex != -1) return arr[minRetIndex]; else return -1; } int main(){ int arr[] = {4, 1, 6, 3, 4, 1, 5, 8}; int n = sizeof(arr) / sizeof(arr[0]); cout<<"The element with repeated occurence is "<<findRepeatingElementArray(arr, n); }
Output
The element with repeated occurence is 4