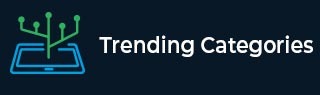
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the first repeated word in a string in Java
To find the first repeated word in a string in Java, the code is as follows −
Example
import java.util.*; public class Demo{ static char repeat_first(char my_str[]){ HashSet<Character> my_hash = new HashSet<>(); for (int i=0; i<=my_str.length-1; i++){ char c = my_str[i]; if (my_hash.contains(c)) return c; else my_hash.add(c); } return '\0'; } public static void main (String[] args){ String my_str = "thisisasampleonlysample"; char[] my_arr = my_str.toCharArray(); System.out.println("The first repeating character in the string is :"); System.out.println(repeat_first(my_arr)); } }
Output
The first repeating character in the string is : I
A class named Demo contains a function named ‘repeat_first’, that takes a character string as a parameter. It creates a new hash set and iterates over the string and checks if the character in the string is equal to a specific character.
If yes, then the character is returned, otherwise, the character is added to the hash set. This way, the second time a word is found, it is added to the hash set, and this becomes the first word, that was in the string more than once. In the main function, the string is defined and a character array is defined. The function ‘repeat_first’ is called on this character array. The relevant array is displayed on the console.
Advertisements