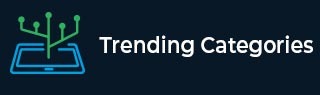
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find the cordinates of the fourth vertex of a rectangle with given 3 vertices in Python
Suppose we have a grid of size Q * P, this grid contains exactly three asterisk '*' and every other cell there is dot '.', where '*' is for a vertex of a rectangle. We have to find the coordinates of the missing vertex. Here we will consider 1-based indexing.
So, if the input is like grid = [ ".*.", "...", "*.*" ], then the output will be [1, 3], this is the missing coordinate.
To solve this, we will follow these steps −
p := number of rows
q := number of columns
row := make a map for all row number, and associated value is 0
col := make a map for all column number, and associated value is 0
for i in range 0 to p, do
for j in range 0 to q, do
if grid[i, j] is same as '*', then
row[i] := row[i] + 1
col[j] := col[j] + 1
for each k,v in row, do
if v is same as 1, then
x_coord := k;
for each k,v in col, do
if v is same as 1, then
y_coord := k;
return(x_coord + 1, y_coord + 1)
Example
Let us see the following implementation to get better understanding −
def get_missing_vertex(grid) : p = len(grid) q = len(grid[0]) row = dict.fromkeys(range(p), 0) col = dict.fromkeys(range(q), 0) for i in range(p) : for j in range(q) : if (grid[i][j] == '*') : row[i] += 1 col[j] += 1 for k,v in row.items() : if (v == 1) : x_coord = k; for k,v in col.items() : if (v == 1) : y_coord = k; return (x_coord + 1, y_coord + 1) grid = [".*.", "...", "*.*"] print(get_missing_vertex(grid))
Input
[".*.", "...", "*.*"]
Output
(1, 3)