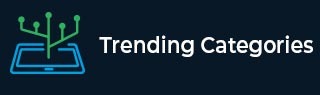
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find sum of frequency of given elements in the list in Python
A given list has many repeated items. We are interested in finding out the sum of the frequency of some such items which are repeated in the list. Below are the approaches how we can achieve this.
With sum
We have two lists. One has the list of values and other has the values whose frequency needs to be checked from the first list. So we create a for loop to count the number of occurrences of the elements from the second list in the first list and then apply the sum function to get the final sum of frequency.
Example
chk_list= ['Mon', 'Tue'] big_list = ['Mon','Tue', 'Wed', 'Mon','Mon','Tue'] # Apply sum res = sum(big_list.count(elem) for elem in chk_list) # Printing output print("Given list to be analysed: \n", big_list) print("Given list to with values to be analysed:\n", chk_list) print("Sum of the frequency: ", res)
Output
Running the above code gives us the following result −
Given list to be analysed: ['Mon', 'Tue', 'Wed', 'Mon', 'Mon', 'Tue'] Given list to with values to be analysed: ['Mon', 'Tue'] Sum of the frequency: 5
With collections.Counter
The Counter function from collections module can get the desired result by applying it to the list whose values have to be analysed while looping through the smaller list which has only the elements whose frequency needs to be established.
Example
from collections import Counter chk_list= ['Mon', 'Tue'] big_list = ['Mon','Tue', 'Wed', 'Mon','Mon','Tue'] # Apply Counter res = sum(Counter(big_list)[x] for x in chk_list) # Printing output print("Given list to be analysed: \n", big_list) print("Given list to with values to be analysed:\n", chk_list) print("Sum of the frequency: ", res)
Output
Running the above code gives us the following result −
Given list to be analysed: ['Mon', 'Tue', 'Wed', 'Mon', 'Mon', 'Tue'] Given list to with values to be analysed: ['Mon', 'Tue'] Sum of the frequency: 5