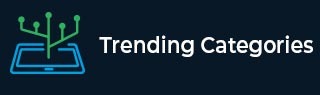
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find smallest permutation of given number in C++
In this problem, we are given a large number N. Our task is to find the smallest permutation of a given number.
Let’s take an example to understand the problem,
Input
N = 4529016
Output
1024569
Solution Approach
A simple solution to the problem is by storing the long integer value to a string. Then we will sort the string which is our result. But if there are any leading zeros, we will shift them after the first non zero value.
Program to illustrate the working of our solution,
Example
#include <bits/stdc++.h> using namespace std; string smallestNumPer(string s) { int len = s.length(); sort(s.begin(), s.end()); int i = 0; while (s[i] == '0') i++; swap(s[0], s[i]); return s; } int main() { string s = "4529016"; cout<<"The number is "<<s<<endl; cout<<"The smallest permutation of the number is "<<smallestNumPer(s); return 0; }
Output
The number is 4529016 The smallest permutation of the number is 1024569
Advertisements