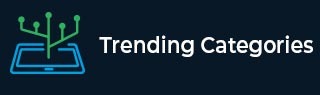
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find original numbers from gcd() every pair in Python
Suppose we have an array A where GCD of every possible pair of elements of another array is given, we have to find the original numbers which are used to compute the given GCD array.
So, if the input is like A = [6, 1, 1, 13], then the output will be [13, 6] as gcd(13, 13) is 13, gcd(13, 6) is 1, gcd(6, 13) is 1, gcd(6, 6) is 6
To solve this, we will follow these steps −
n := size of A
sort array A in descending order
occurrence := an array of size A[0] and fill with 0
for i in range 0 to n, do
occurrence[A[i]] := occurrence[A[i]] + 1
size := integer of square root of n
res := an array of size same as size of A and fill with 0
l := 0
for i in range 0 to n, do
if occurrence[A[i]] > 0, then
res[l] := A[i]
occurrence[res[l]] := occurrence[res[l]] - 1
l := l + 1
for j in range 0 to l, do
if i is not same as j, then
g := gcd(A[i], res[j])
occurrence[g] := occurrence[g] - 2
return res[from index 0 to size]
Example
Let us see the following implementation to get better understanding −
from math import sqrt, gcd def get_actual_array(A): n = len(A) A.sort(reverse = True) occurrence = [0 for i in range(A[0] + 1)] for i in range(n): occurrence[A[i]] += 1 size = int(sqrt(n)) res = [0 for i in range(len(A))] l = 0 for i in range(n): if (occurrence[A[i]] > 0): res[l] = A[i] occurrence[res[l]] -= 1 l += 1 for j in range(l): if (i != j): g = gcd(A[i], res[j]) occurrence[g] -= 2 return res[:size] A = [6, 1, 1, 13] print(get_actual_array(A))
Input
[6, 1, 1, 13]
Output
[13, 6]