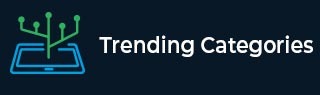
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find numbers with K odd divisors in a given range in C++
In this problem, we are given three integer values, L, R, and k. Our task is to find numbers with K odd divisors in a given range. We will be finding the count of numbers in the range [L, R] that have exactly k divisors.
We will be counting the 1 and the number itself as a divisor.
Let’s take an example to understand the problem,
Input
a = 3, b = 10, k = 3
Output
2
Explanation
Numbers with exactly 3 divisors within the range 3 to 10 are 4 : divisors = 1, 2, 4 9 : divisors = 1, 3, 9
Solution Approach
A simple solution to the problem is by counting the k divisors. So,for k to be an odd number (as depicted in problem), the number has to be a perfect square. So, we will count the number of divisors for only the perfect square number (this will save compilation time). And if the count of divisors in k, we will add 1 to the number count.
Program to illustrate the working of our solution,
Example
#include<bits/stdc++.h> using namespace std; bool isPerfectSquare(int n) { int s = sqrt(n); return (s*s == n); } int countDivisors(int n) { int divisors = 0; for (int i=1; i<=sqrt(n)+1; i++) { if (n%i==0) { divisors++; if (n/i != i) divisors ++; } } return divisors; } int countNumberKDivisors(int a,int b,int k) { int numberCount = 0; for (int i=a; i<=b; i++) { if (isPerfectSquare(i)) if (countDivisors(i) == k) numberCount++; } return numberCount; } int main() { int a = 3, b = 10, k = 3; cout<<"The count of numbers with K odd divisors is "<<countNumberKDivisors(a, b, k); return 0; }
Output
The count of numbers with K odd divisors is 2
Advertisements