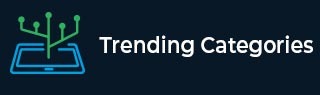
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find number of pairs (x, y) in an array such that x^y > y^x in C++
Suppose we have two arrays X and Y of positive integers. Find the number of pairs such that x^y > y^x, where x is an element of X and y is an element of Y. Suppose the X = [2, 1, 6], and Y = [1, 5], then output will be 3. As there are three pairs, these are (2, 1), (2, 5) and (6, 1)
We can solve this in an efficient way. The logic is simple, it will be when y > x then x^y > y^x with some exceptions. So this is the trick.
Sort the array Y
for each element x in X, we have to find the index of the smallest number that is greater than x in Y. We will use the binary search to do so. Otherwise we can use the upper_bound() function also.
All the number after the found index satisfy the relation so just add that into the count.
Example
#include <iostream> #include <algorithm> using namespace std; int count(int x, int Y[], int n, int no_of_y[]) { if (x == 0) return 0; if (x == 1) return no_of_y[0]; int* index = upper_bound(Y, Y + n, x); int ans = (Y + n) - index; ans += (no_of_y[0] + no_of_y[1]); if (x == 2) ans -= (no_of_y[3] + no_of_y[4]); if (x == 3) ans += no_of_y[2]; return ans; } int howManyPairs(int X[], int Y[], int m, int n) { int no_of_y[5] = {0}; for (int i = 0; i < n; i++) if (Y[i] < 5) no_of_y[Y[i]]++; sort(Y, Y + n); int total_pairs = 0; for (int i=0; i< m; i++) total_pairs += count(X[i], Y, n, no_of_y); return total_pairs; } int main() { int X[] = {2, 1, 6}; int Y[] = {1, 5}; int m = sizeof(X)/sizeof(X[0]); int n = sizeof(Y)/sizeof(Y[0]); cout << "Total pair count: " << howManyPairs(X, Y, m, n); }
Output
Total pair count: 3
Advertisements