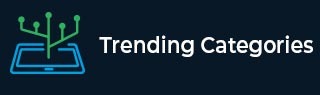
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find Maximum Element in Each Row of a Matrix in Java
In Java, Array is an object. It is a non-primitive data type which stores values of similar data type. The matrix in java is nothing but a multi-dimensional array which represents multiple rows and columns.
Here we have given a matrix which contains set of elements and as per the problem statement we have to find out the maximum element in each row of that matrix.
Let’s deep dive into this article, to know how it can be done by using Java programming language.
To show you some instances
Instance-1
Given matrix =
21 22 23 24 25 26 27 28 29
Maximum element in each row: 23, 26 and 29
Instance-2
Given matrix =
121 222 243 432 124 245 256 657 237 258 229 345 176 453 756 343
Maximum element in each row: 432, 657, 345 and 756
Instance-3
Given matrix =
1 2 3 4 5 6 7 8 9
Maximum element in each row: 3, 6 and 9
Algorithm
Algorithm-1: (By Using for Loop)
Step-1 − Use two nested for loops to iterate through the matrix and find the maximum element in each row.
Step-2 − The outer loop iterates through each row of the matrix, while the inner loop iterates through each element in the row.
Step-3 − The maximum element in the row is found by using the Math.max method, which returns the larger of two values.
Step-4 − The result is stored in an array, which is returned at the end.
Algorithm-2: (Using Java Stream)
Step-1 − Use Java's stream API to find the maximum element in each row.
Step-2 − The IntStream.range method is used to create a stream of integers from 0 to the number of rows in the matrix.
Step-3 − The map method is used to apply the function IntStream.of(matrix[i]).max().getAsInt() to each integer in the stream.
Step-4 − This function returns the maximum element in the row matrix[i] as an integer.
Step-5 − The result is stored in an array, which is returned at the end.
Syntax
The Matrix.length() method in Java returns the length of the given matrix.
Below refers to the syntax of it −
inputMatrix.lenght
where, ‘inputMatrix’ refers to the given matrix.
IntStream.range() is a method in Java that generates a sequential stream of integers from startInclusive to endExclusive - 1.
IntStream.range(startInclusive, endExclusive)
It can be used to perform operations on a set of integers. For example, in the second code, it is used to loop through the rows and columns of the matrix.
Multiple Approaches
We have provided the solution in different approaches.
By Using for Loop
By Using Stream Method
Let’s see the program along with its output one by one.
Approach-1: By Using Nested for Loop
In this approach, matrix elements will be initialized in the program. Then calls a user defined method by passing the matrix as parameter and inside method as per the algorithm using a for loop find maximum element in each row of that matrix.
Example
import java.util.Arrays; public class Main { public static void main(String[] args) { int[][] inputMatrix = {{12, 12, 3}, {74, 65, 64}, {57, 28, 49}}; System.out.println("Max element in each row: "); int[] result = maxInEachRow(inputMatrix); System.out.println(Arrays.toString(result)); } // Method to find the maximum element in each row of the matrix public static int[] maxInEachRow(int[][] mat) { // Array to store the result int[] result = new int[mat.length]; // Outer loop to iterate through each row of the matrix for (int i = 0; i < mat.length; i++) { // Initialize max to the minimum value of int int max = Integer.MIN_VALUE; // Inner loop to iterate through each element in the row for (int j = 0; j < mat[i].length; j++) { // Update max with the maximum of the current value and the next element max = Math.max(max, mat[i][j]); } // Store the result in the array result[i] = max; } return result; } }
Output
Max element in each row: [12, 74, 57]
Approach-2: By Using Stream Method
In this approach, matrix elements will be initialized in the program. Then calls a user defined method by passing the matrix as parameter and inside method as per the algorithm using stream method find maximum element in each row of that matrix.
Example
import java.util.Arrays; import java.util.stream.IntStream; public class Main { public static void main(String[] args) { int[][] matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}; System.out.println("Max element in each row: "); int[] result = maxInEachRow(matrix); System.out.println(Arrays.toString(result)); } // Method to find the maximum element in each row of the matrix using streams public static int[] maxInEachRow(int[][] matrix){ return IntStream.range(0, matrix.length) // Get the maximum element in the row .map(i -> IntStream.of(matrix[i]).max().getAsInt()) // Convert the stream to an array .toArray(); } }
Output
Max element in each row: [3, 6, 9]
In this article, we explored different approaches to find the maximum element in each row of the matrix by using Java programming language.