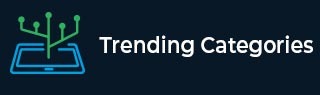
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find length of longest subsequence of one string which is substring of another string in C++
Suppose, we have two strings X and Y, and we have to find the length of longest subsequence of string X, which is substring in sequence Y. So if X = “ABCD” and Y = “BACDBDCD”, then output will be 3. As “ACD” is the longest sub-sequence of X, which is substring of Y.
Here we will use the dynamic programming approach to solve this problem. So if the length of X is n, and length of Y is m, then create DP array of order (m+1)x(n+1). Value of DP[i, j] is maximum length of subsequence of X[0…j], which is substring of Y[0…i]. Now for each cell of DP, it will follow like below:
- for i in range 1 to m:
- for j in range 1 to n
- when X[i – 1] is same as Y[j – i], then DP[i, j] := 1 + DP[i – 1, j – 1]
- Otherwise DP[i, j] := 1 + DP[i, j – 1]
- for j in range 1 to n
And finally the length of longest subsequence of x, which is substring of y is max(DP[i, n]), where 1 <= i <= m.
Example
#include<iostream> #define MAX 100 using namespace std; int maxSubLength(string x, string y) { int table[MAX][MAX]; int n = x.length(); int m = y.length(); for (int i = 0; i <= m; i++) for (int j = 0; j <= n; j++) table[i][j] = 0; for (int i = 1; i <= m; i++) { for (int j = 1; j <= n; j++) { if (x[j - 1] == y[i - 1]) table[i][j] = 1 + table[i - 1][j - 1]; else table[i][j] = table[i][j - 1]; } } int ans = 0; for (int i = 1; i <= m; i++) ans = max(ans, table[i][n]); return ans; } int main() { string x = "ABCD"; string y = "BACDBDCD"; cout << "Length of Maximum subsequence substring is: " << maxSubLength(x, y); }
Output
Length of Maximum subsequence substring is: 3
Advertisements