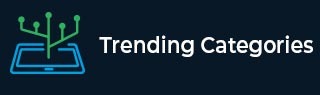
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find Largest & Smallest Element in Each Column of a Matrix in Java?
In Java, Array is an object. It is a non-primitive data type which stores values of similar data type. The matrix in java is nothing but a multi-dimensional array which represents multiple rows and columns.
Here we have given a matrix which contains set of elements and as per the problem statement we have to find out the largest and smallest element in each column of that matrix.
Let’s deep dive into this article, to know how it can be done by using Java programming language.
To show you some instances
Instance-1
Given matrix =
21 22 23 24 25 26 27 28 29
The largest element in column 1 is 27
The largest element in column 2 is 28
The largest element in column 3 is 29
The smallest element in column 1 is 21
The smallest element in column 2 is 22
The smallest element in column 3 is 23
Instance-2
Given matrix =
121 222 243 432 124 245 256 657 237 258 229 345 176 453 756 343
The largest element in column 2 is 453
The largest element in column 3 is 756
The largest element in column 4 is 657
The smallest element in column 1 is 121
The smallest element in column 2 is 222
The smallest element in column 3 is 229
The smallest element in column 4 is 343
Instance-3
Given matrix =
1 2 3 4 5 6 7 8 9
The largest element in column 1 is 7
The largest element in column 2 is 8
The largest element in column 3 is 9
The smallest element in column 1 is 1
The smallest element in column 2 is 2
The smallest element in column 3 is 3
Algorithm
Algorithm-1: (By Using Nested for Loop)
Step-1 − Define a 2D matrix.
Step-2 − Get the number of rows and columns.
Step-3 − Find the largest elements in each column using a nested for loop. Compare each element in the column to the current maximum, updating the maximum if a larger element is found.
Step-4 − Find the smallest elements in each column using another nested for loop. Compare each element in the column to the current minimum, updating the minimum if a smaller element is found.
Step-5 − Print the result as output.
Algorithm-2: (By using Stream API)
Step-1 − Define a 2D matrix.
Step-2 − Get the number of columns.
Step-3 − Find the largest elements in each column using a for loop and the Java stream API. Map the elements in each column to a stream of integers, and find the maximum value in that stream.
Step-4 − Find the smallest elements in each column using another for loop and the Java stream API. Map the elements in each column to a stream of integers, and find the minimum value in that stream.
Step-5 − Print the result as output.
Syntax
The Matrix.length() method in Java returns the length of the given matrix.
Below refers to the syntax of it −
inputMatrix.lenght
where, ‘inputMatrix’ refers to the given matrix.
Arrays.stream(matrix) converts the two-dimensional integer array matrix into a stream of arrays, where each array in the stream represents a row in the matrix.
Arrays.stream(matrix)
.mapToInt(row -> row[column]) maps each array (row) in the stream to an integer value corresponding to the element at the specified column index.
.mapToInt(row -> row[column])
.max() returns the maximum element in the stream.
.max()
.min() returns the minimum element in the stream.
.min()
Multiple Approaches
We have provided the solution in different approaches.
By Using Nested For Loop
By Using Stream API
Let’s see the program along with its output one by one.
Approach-1: By Using Nested for Loop
In this approach, matrix elements will be initialized in the program. Then as per the algorithm by using nested for loop find largest and smallest element in each column of that matrix.
Example
public class Main { public static void main(String[] args) { int[][] inputMatrix = { {101, 15, 121}, {210, 115, 71}, {81, 215, 118} }; int r = inputMatrix.length; int c = inputMatrix[0].length; //loop to find the largest element in each column for (int x = 0; x < c; x++) { int maxm = inputMatrix[0][x]; for (int y = 1; y < r; y++) { if (inputMatrix[y][x] > maxm) { maxm = inputMatrix[y][x]; } } System.out.println("The largest element in column " + (x+1) + " is " + maxm); } //loop to find the smallest elements in each column for (int x = 0; x < c; x++) { int mimm = inputMatrix[0][x]; for (int y = 1; y < r; y++) { if (inputMatrix[y][x] < mimm) { mimm = inputMatrix[y][x]; } } System.out.println("The smallest element in column " + (x+1) + " is " + mimm); } } }
Output
The largest element in column 1 is 210 The largest element in column 2 is 215 The largest element in column 3 is 121 The smallest element in column 1 is 81 The smallest element in column 2 is 15 The smallest element in column 3 is 71
Approach-2: By Using Dynamic Initialization of Matrix
In this approach, matrix elements will be initialized in the program. Then as per the algorithm by using Java streams find largest and smallest element in each column of that matrix.
Example
import java.util.Arrays; public class Main { public static void main(String[] args) { // Define the matrix int[][] matrix = { {1, 5, 3}, {2, 7, 4}, {9, 6, 8} }; // Get the number of columns int numCols = matrix[0].length; // Iterate over each column for (int i = 0; i < numCols; i++) { // Create a final variable to hold the current column number final int column = i; // Use Java streams to find the largest element in the column int max = Arrays.stream(matrix) .mapToInt(row -> row[column]) .max() .getAsInt(); // Use Java streams to find the smallest element in the column int min = Arrays.stream(matrix) .mapToInt(row -> row[column]) .min() .getAsInt(); // Output the results to the console System.out.println("---------------"); System.out.println("Column " + (i + 1) + ":"); System.out.println("---------------"); System.out.println("Largest element: " + max); System.out.println("Smallest element: " + min); } } }
Output
--------------- Column 1: --------------- Largest element: 9 Smallest element: 1 --------------- Column 2: --------------- Largest element: 7 Smallest element: 5 --------------- Column 3: --------------- Largest element: 8 Smallest element: 3
In this article, we explored different approaches to find largest and smallest element in each column of the matrix by using Java programming language.