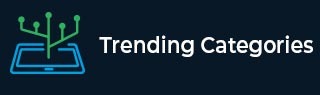
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find Indexs of 0 to be replaced with 1 to get longest continuous sequence of 1s in a binary array - Set-2 in C++
Concept
With respect of a given array of 0s and 1s, determine the position of 0 to be replaced with 1 to get maximum continuous sequence of 1s. In this case, expected time complexity is O(n) and auxiliary space is O(1).
Input
arr[] = {1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 0, 1, 1}
Output
Index 10
Let array index starts from 0, replacing 0 with 1 at
index 10 causes the longest continuous sequence of 1s.
Input
arr[] = {1, 1, 1, 1, 1, 0}
Output
Index 5
Method
Using count of ones on both sides of zero −
Now,the concept is to count number of ones on both sides of each zero. Here, the required index is treated as the index of zero having maximum number of ones around it. The following variables are implemented regarding this purpose −
- leftCnt − It is used to store count of ones on left side of current element zero under consideration.
- rightCnt − It is used to store count of ones on right side of current element zero under consideration.
- maxIndex − It is treated as index of zero with maximum number of ones around it.
- lastInd − It is treated as index of last zero element seen
- maxCnt − It is treated as count of ones if zero at index maxInd is replaced by one.
Now procedure details are given below −
Maintain incrementing rightCnt until one is present in input array. Assume next zero is present at index i.
Verify whether this zero element is first zero element or not. Now it is treated as first zero element if lastInd does not hold any valid index value.
So in that case updating lastInd is done with i. At present the value of rightCnt is number of zeroes on left side of this zero.
As a result of this leftCnt is equal to rightCnt and then again determine value of rightCnt. It has been seen that if current zero element is not first zero, then number of ones around zero present at index lastInd is provided by leftCnt + rightCnt.
Now maxCnt will accept value leftCnt + rightCnt + 1 and maxIndex = lastInd if this value is smaller than value currently held by maxCnt.
At present rightCnt will become leftCnt for zero at index i and lastInd will be equal to i. Now again determine value of rightCnt, compare number of ones with maxCnt and update maxCnt and maxIndex accordingly.
We have to repeat this procedure for each subsequent zero element of the array.
It has been observed that lastInd stores the index of zero for which current leftCnt and rightCnt are computed.
Finally, the required index of zero to be replaced with one is stored in maxIndex.
Example
// C++ program to find index of zero // to be replaced by one to get longest // continuous sequence of ones. #include <bits/stdc++.h> using namespace std; // Used to returns index of 0 to be replaced // with 1 to get longest continuous // sequence of 1s. If there is no 0 // in array, then it returns -1. int maxOnesIndex(bool arr1[], int n1){ int i = 0; // Used to store count of ones on left // side of current element zero int leftCnt1 = 0; // Used to store count of ones on right // side of current element zero int rightCnt1 = 0; // Shows index of zero with maximum number // of ones around it. int maxIndex1 = -1; // Shows index of last zero element seen int lastInd1 = -1; // Shows count of ones if zero at index // maxInd1 is replaced by one. int maxCnt1 = 0; while (i < n1) { // Used to keep incrementing count until // current element is 1. if (arr1[i]) { rightCnt1++; } else { // It has been observed that if current zero element // is not first zero element, // then count number of ones // obtained by replacing zero at // index lastInd. Update maxCnt // and maxIndex if required. if (lastInd1 != -1) { if (rightCnt1 + leftCnt1 + 1 > maxCnt1) { maxCnt1 = leftCnt1 + rightCnt1 + 1; maxIndex1 = lastInd1; } } lastInd1 = i; leftCnt1 = rightCnt1; rightCnt1 = 0; } i++; } // Determine number of ones in continuous // sequence when last zero element is // replaced by one. if (lastInd1 != -1) { if (leftCnt1 + rightCnt1 + 1 > maxCnt1) { maxCnt1 = leftCnt1 + rightCnt1 + 1; maxIndex1 = lastInd1; } } return maxIndex1; } // Driver function int main(){ bool arr1[] = { 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 0, 1, 1 }; // bool arr1[] = {1, 1, 1, 1, 1, 0}; int n1 = sizeof(arr1) / sizeof(arr1[0]); cout << "Index of 0 to be replaced is " << maxOnesIndex(arr1, n1); return 0; }
Output
Index of 0 to be replaced is 10