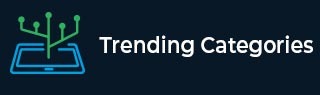
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find if two people ever meet after same number of jumps in C++
In this problem, we are given four integers denoting the starting points and jumps taken by each in the race. Our task is to find if two people ever meet after same number of jumps.
Problem Description: Here, we need to check if two persons starting at points p1 and p2 taking jumps j1 and j2 will be at some point in the path or not.
Let’s take an example to understand the problem,
Input: p1 = 5, p2 = 9, j1 = 4, j2 = 2
Output: Yes
Explanation:
After first jump, p1 = 9, p2 = 11
After second jump, p1 = 13, p2 = 13
Solution Approach:
For meeting at some point both the people will have to jump different distances. Here are some conditions that are to be meet to check if the meet of people is possible,
If p1 > p2 then s1 needs to be less than s2.
And ( (p2 - p1) % (s1 - s2) ) == 0
Then the meeting is possible otherwise not possible.
Program to illustrate the working of our solution,
Example
#include<iostream> using namespace std; bool WillMeet(int p1, int j1, int p2, int j2){ return ( (j1 > j2 && ( ((p2 - p1) % (j1 - j2)) == 0)) || (j2 > j1 && ( ((p1 - p2) % (j2 - j1)) == 0)) ); } int main() { int p1 = 5, j1 = 4, p2 = 9, j2 = 2; if(WillMeet(p1, j1, p2, j2)) cout<<"Both will meet at some point"; else cout<<"Both will not meet at any point"; return 0; }
Output
Both will meet at some point