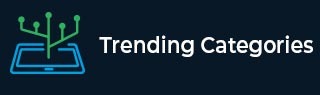
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find frequency of each element in a limited range array in less than O(n) time in C++
Suppose we have an array of integers. The array is A, and the size is n. Our task is to find the frequency of all elements in the array less than O(n) time. The size of the elements must be less than one value say M. Here we will use the binary search approach. Here we will recursively divide the array into two parts if the end elements are different, if both its end elements are the same, it means all elements in the array are the same as the array is already sorted.
Example
#include<iostream> #include<vector> using namespace std; void calculateFreq(int arr[], int left, int right, vector<int>& frequency) { if (arr[left] == arr[right]) frequency[arr[left]] += right - left + 1; else { int mid = (left + right) / 2; calculateFreq(arr, left, mid, frequency); calculateFreq(arr, mid + 1, right, frequency); } } void getAllFrequency(int arr[], int n) { vector<int> frequency(arr[n - 1] + 1, 0); calculateFreq(arr, 0, n - 1, frequency); for (int i = 0; i <= arr[n - 1]; i++) if (frequency[i] != 0) cout << "Frequency of element " << i << " is " << frequency[i] << endl; } int main() { int arr[] = { 10, 10, 10, 20, 30, 30, 50, 50, 80, 80, 80, 90, 90, 99 }; int n = sizeof(arr) / sizeof(arr[0]); getAllFrequency(arr, n); }
Output
Frequency of element 10 is 3 Frequency of element 20 is 1 Frequency of element 30 is 2 Frequency of element 50 is 2 Frequency of element 80 is 3 Frequency of element 90 is 2 Frequency of element 99 is 1
Advertisements