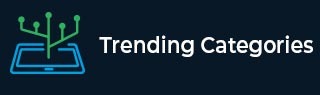
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find first undeleted integer from K to N in given unconnected Graph after Performing Q queries
Introduction
Finding the primary undeleted integer from a given extend in a detached graph after performing multiple queries may be a challenging issue in graph theory. In this article, we investigate the errand of distinguishing the primary undeleted numbers and give two approaches to fathom it utilizing C++. Each approach offers a diverse point of view and utilizes distinctive calculations and data structures. The problem includes developing a graph, checking certain nodes as deleted, and after that deciding the primary undeleted numbers inside an indicated extend. The graph represents associations between nodes, and the deleted nodes are those that have been expelled or checked as invalid.
Approach 1: Depth-First Search (DFS)
In this approach, we utilize a depth-first search traversal of the graph. We begin from a given node and investigate its neighbor's recursively. We check the deleted nodes and proceed the traversal until we discover the primary undeleted numbers inside the required extend.
Algorithm
Step 1 − Build the graph.
Step 2 − Check the deleted nodes.
Step 3 − Execute a depth-first look (DFS) calculation to navigate the graph.
Step 4 − During the DFS traversal, check in the event that each node is deleted or not.
Step 5 − Return the primary undeleted numbers experienced.
Example
#include <iostream> #include <vector> using namespace std; vector<bool> traverse; vector<bool> deleted; vector<vector<int> > tg; void dfs(int node, int& result) { traverse[node] = true; if (!deleted[node]) { result = node; return; } for (int i = 0; i < tg[node].size(); i++) { int neighbor = tg[node][i]; if (!traverse[neighbor]) { dfs(neighbor, result); if (result != -1) return; } } } int findFirstUndeleted(int K, int N) { traverse.assign(N + 1, false); int result = -1; for (int i = K; i <= N; i++) { if (!traverse[i]) { dfs(i, result); if (result != -1) return result; } } return result; } int main() { int K = 1; int N = 10; // Construct the graph tg.resize(N + 1); // Add edges to the graph // ... // insert data at the end of the graph // ... tg[1].push_back(2); tg[1].push_back(1); tg[1].push_back(3); tg[1].push_back(2); tg[1].push_back(4); // Set deleted nodes deleted.resize(N + 1, false); deleted[1] = true; deleted[1] = true; deleted[1] = true; int firstUndeleted = findFirstUndeleted(K, N); cout << "First undeleted integer: " << firstUndeleted << endl; return 0; }
Output
First undeleted integer: 2
Approach 2: Breadth- First Search (BFS)
In this approach, we utilize a breadth-first search traversal of the graph. We begin from a given node and investigate its neighbors iteratively employing a queue. We stamp the deleted nodes and proceed the traversal until we discover the primary undeleted numbers inside the desired run.
Algorithm
Step 1 − Build the chart.
Step 2 − Check the erased hubs.
Step 3 − Actualize a breadth-first look (BFS) calculation to navigate the chart.
Step 4 − Amid the BFS traversal, check in case each hub is erased or not.
Step 5 − Return the primary undeleted numbers experienced.
Example
#include <iostream> #include <vector> #include <queue> using namespace std; vector<bool> visited; vector<bool> deleted; vector<vector<int> > graph; int findFirstUndeleted(int K, int N) { visited.assign(N + 1, false); queue<int> q; int result = -1; for (int i = K; i <= N; i++) { if (!visited[i]) { q.push(i); visited[i] = true; while (!q.empty()) { int node = q.front(); q.pop(); if (!deleted[node]) { result = node; break; } for (int j = 0; j < graph[node].size(); j++) { int neighbor = graph[node][j]; if (!visited[neighbor]) { q.push(neighbor); visited[neighbor] = true; } } } if (result != -1) break; } } return result; } int main() { int K = 1; int N = 10; // Construct the graph graph.resize(N + 1); // Add edges to the graph // ... // insert nodes // ... graph[1].push_back(2); graph[2].push_back(1); graph[2].push_back(3); graph[3].push_back(2); graph[3].push_back(4); graph[4].push_back(3); graph[4].push_back(5); graph[5].push_back(4); graph[6].push_back(7); graph[7].push_back(6); graph[8].push_back(9); graph[9].push_back(8); graph[10].push_back(5); // Set deleted nodes deleted.resize(N + 1, false); deleted[2] = true; deleted[5] = true; deleted[7] = true; int firstUndeleted = findFirstUndeleted(K, N); cout << "First undeleted integer: " << firstUndeleted << endl; return 0; }
Output
First undeleted integer: 1
Conclusion
In this article, we investigated two approaches to discover the primary undeleted numbers from a given run in a detached graph. Each approach advertised a one of a kind viewpoint and utilized distinctive calculations and information structures. Approach 1 utilized a depth-first search (DFS) traversal, Approach 2 utilized a breadth-first search (BFS) traversal. These approaches showcased diverse procedures to handle the issue, and depending on the characteristics of the graph and the prerequisites of the errand, one approach may be more reasonable than the others. By understanding these approaches and their comparing executions in C++, users can pick up bits of knowledge into fathoming comparative graph-based issues proficiently.