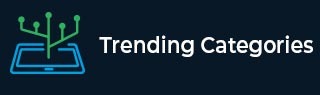
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find First element in AP which is multiple of given Prime in C++
Concept
With respect of given first term (A) and common difference (d) of an Arithmetic Progression, and a prime number (P), our task is to determine the position of the first element in the given AP which is treated as a multiple of the given prime number P.
Input
A = 3, d = 4, P = 5
Output
3
Explanation
The fourth term of the given AP is a multiple of prime number 5.
First Term = 3
Second Term = 3+4 = 7
Third Term = 3+2*4 = 11
Fourth Term = 3+3*4 = 15
Method
Assume the term be AN. As a result of this,
AN = (A + (N-1)*d)
So, it is given that AN is a multiple of P. As aresult of this,
A + (N-1)*d = l*P
Here, l is a constant.
So assume A be (A % P) and d be (d % P). Now, we have (N-1)*d = (l*P – A).
With the help of adding and subtracting P on RHS, we obtain −
(N-1)*d = P(l-1) + (P-A),
In this case, P-A is treated as a non-negative number
(because A is replaced by A%P which is smaller than P) At last taking mod on both sides −
((N-1)*d)%P = (P-A)%P or, ((N-1)d)%P = P-A
Assume calculate a Y < P, so that (d*Y)%P = 1. Now, this Y is termed as the inverse modulo of d with respect to P.
Finally answer N is −
((Y*(P-A)) % P) + 1.
Example
#include <bits/stdc++.h> using namespace std; // Shows iterative Function to calculate // (x1^y1)%p1 in O(log y1) */ int power(int x1, int y1, int p1){ // Used to initialize result int res1 = 1; // Used to update x if it is more than or // equal to p x1 = x1 % p1; while (y1 > 0) { // It has been seen that if y1 is odd, multiply x1 with result if (y1 & 1) res1 = (res1 * x1) % p1; // y1 must be even now y1 = y1 >> 1; // y1 = y1/2 x1 = (x1 * x1) % p1; } return res1; } // Shows function to find nearest element in common int NearestElement1(int A, int d, int P){ // Shows base conditions if (A == 0) return 0; else if (d == 0) return -1; else { int Y = power(d, P - 2, P); return (Y * (P - A)) % P; } } // Driver code int main(){ int A = 3, d = 4, P = 5; // Used to module both A and d A %= P; d %= P; // Shows function call cout << NearestElement1(A, d, P); return 0; }
Output
3