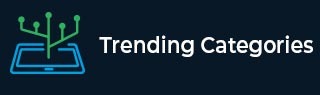
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Find all subarrays with sum equal to number? JavaScript (Sliding Window Algorithm)
We are given an array of numbers and a number; our job is to write a function that returns an array of all the sub arrays which add up to the number provided as second argument.
For example −
const arr = [23, 5, 1, 34, 12, 67, 9, 31, 6, 7, 27]; const sum = 40; console.log(requiredSum(arr, sum));
Should output the following array −
[ [ 5, 1, 34 ], [ 9, 31 ], [ 6, 7, 27 ] ]
Because each of these 3 sub arrays add up to 40.
The Sliding Window Algorithm (Linear Time)
This algorithm is mostly used when we are required to find subarrays within an array / substring within a string that satisfy certain criteria. And this very problem is a perfect candidate for the sliding window algorithm.
Sliding window algorithm is just what its name suggests, we create a window which is nothing but a subarray of the original array. This window tries to gain stability by increasing or decreasing.
By stability we mean the condition specified in the problem (like adding up to a specific number here). Once it becomes stable we record the window and continue with sliding it. Generally, in 90% of the problems, we start the window from left and keep sliding it until its end reaches the end of the array / string.
Let’s look at the code to make ourselves more familiar with the Sliding Windows algorithm.
Example
const arr = [23, 5, 1, 34, 12, 67, 9, 31, 6, 7, 27]; const sum = 40; const findSub = (arr, sum) => { const required = []; for(let start = 0, end = 0, s = 0; end <= arr.length || s > sum ; ){ if(s < sum){ s += arr[end]; end++; }else if(s > sum){ s -= arr[start]; start++; }else{ required.push(arr.slice(start, end)); s -= arr[start]; s += arr[end]; start++; end++; }; }; return required; }; console.log(findSub(arr, sum));
The start and end variables denote the starting and ending positions of the window at different points.
Initially both started at 0, then we increased the size of the window if the required sum was less than the given sum, decreased the window size if it was greater and if at any point both the sum equaled, we pushed that subarray into the required array. And moved the window towards right by unit distance.
Output
The output of this code in the console will be −
[ [ 5, 1, 34 ], [ 9, 31 ], [ 6, 7, 27 ] ]