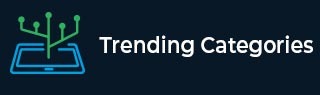
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Filter away object in array with null values JavaScript
Let’s say, we have an array of objects about some employees of a company. But the array contains some bad data i.e., key pointing to empty strings or false values. Our job is to write a function that takes in the array and away the objects that have null or undefined or empty string value for the name key and return the new object.
The array of objects are like this −
let data = [{ "name": "Ramesh Dhiman", "age": 67, "experience": 45, "description": "" }, { "name": "", "age": 31, "experience": 9, "description": "" }, { "name": "Kunal Dhiman", "age": 27, "experience": 7, "description": "" }, { "name": "Raman Kumar", "age": 34, "experience": 10, "description": "" }, { "name": "", "age": 41, "experience": 19, "description": "" } ]
Let’s write the code for this function −
Example
let data = [{ "name": "Ramesh Dhiman", "age": 67, "experience": 45, "description": "" }, { "name": "", "age": 31, "experience": 9, "description": "" }, { "name": "Kunal Dhiman", "age": 27, "experience": 7, "description": "" }, { "name": "Raman Kumar", "age": 34, "experience": 10, "description": "" }, { "name": "", "age": 41, "experience": 19, "description": "" } ] const filterUnwanted = (arr) => { const required = arr.filter(el => { return el.name; }); return required; }; console.log(filterUnwanted(data));
Output
The output in the console will be −
[ { name: 'Ramesh Dhiman', age: 67, experience: 45, description: '' }, { name: 'Kunal Dhiman', age: 27, experience: 7, description: '' }, { name: 'Raman Kumar', age: 34, experience: 10, description: '' } ]
Advertisements