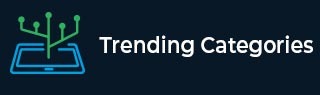
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
FabricJS – How to remove the current object transform in a cloned image?
In this tutorial, we are going to learn how to remove the current object transform (scale, angle, flip and skew) in a cloned image using FabricJS. We can create an Image object by creating an instance of fabric.Image. Since it is one of the basic elements of FabricJS, we can also easily customize it by applying properties like angle, opacity etc. In order to remove the current object transform in a cloned image, we use the withoutTransform property.
Syntax
cloneAsImage( callback: function, { withoutTransform: Boolean }: Object): fabric.Object
Parameters
callback (optional) − This parameter is a function which is to be invoked with a cloned image instance as the first argument.
options (optional) − This parameter is an optional Object which provides additional customizations to our clone image. Using this parameter we can set a multiplier, crop the clone image, remove the current object transform or a lot of other properties can be changed of which withoutTransform is a property.
Options Keys
withoutTransform − This property accepts a Boolean value which determines whether the current object transform is to be removed or not. This property is optional.
Using the withoutTransform property and passing it a ‘true’ value
Example
Let’s see a code example of how the cloned Image object appears when the withoutTransform property is used and a ‘true’ value is passed to it. The image object already has skew transformation. However since we are passing a ‘true’ value to withoutTransform property, that object transformation will be disabled and our clone image will no longer possess skew transformation.
<!DOCTYPE html> <html> <head> <!-- Adding the Fabric JS Library--> <script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/510/fabric.min.js"></script> </head> <body> <h2>Using the withoutTransform property and passing it a ‘true’ value</h2> <p>You can see that clone image does not have skew transformation</p> <canvas id="canvas"></canvas> <img src="https://www.tutorialspoint.com/images/logo.png" id="img1" style="display: none" /> <script> // Initiate a canvas instance var canvas = new fabric.Canvas("canvas"); canvas.setWidth(document.body.scrollWidth); canvas.setHeight(250); // Initiating the image element var imageElement = document.getElementById("img1"); // Initiate a shadow object var shadow = new fabric.Shadow({ color: "#308080", blur: 3, }); // Initiate an Image object var image = new fabric.Image(imageElement, { top: 50, left: 110, skewX: 20, shadow: shadow, }); // Using cloneAsImage method image.cloneAsImage( function (Img) { Img.set("top", 150); Img.set("left", 150); canvas.add(Img); }, { withoutTransform: true, } ); </script> </body> </html>
Using the withoutTransform property and passing it a ‘false’ value
Example
In this example, we have used the withoutTransform property and passed it a ‘false’ value. This means that whichever object transform our image object has, its clone image will also contain those. Thus, since our image object has a horizontal angle of skew(skewX) of 20 and withoutTransform has been passed a ‘false’ value, the clone image object will also have the same.
<!DOCTYPE html> <html> <head> <!-- Adding the Fabric JS Library--> <script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/510/fabric.min.js"></script> </head> <body> <h2>Using the withoutTransform property and passing it a ‘false’ value</h2> <p>You can see that clone image contains skew transformation</p> <canvas id="canvas"></canvas> <img src="https://www.tutorialspoint.com/images/logo.png" id="img1" style="display: none" /> <script> // Initiate a canvas instance var canvas = new fabric.Canvas("canvas"); canvas.setWidth(document.body.scrollWidth); canvas.setHeight(250); // Initiating the image element var imageElement = document.getElementById("img1"); // Initiate a shadow object var shadow = new fabric.Shadow({ color: "#308080", blur: 3, }); // Initiate an Image object var image = new fabric.Image(imageElement, { top: 50, left: 110, skewX: 20, shadow: shadow, }); // Using cloneAsImage method image.cloneAsImage( function (Img) { Img.set("top", 150); Img.set("left", 150); canvas.add(Img); }, { withoutTransform: false, } ); </script> </body> </html>