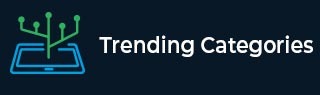
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Extract a data frame column values as a vector by matching a vector in R.
To extract a data frame column values as a vector by matching a vector in R, we can use subsetting with %in% operator.
For Example, if we have a data frame called df having a column say C and a vector V and we want to extract values in C if they match with V then we can use the command given below −
df[df$C %in% V,"C"]
Example 1
Following snippet creates a sample data frame and vector −
x1<-rpois(20,5) x2<-rpois(20,2) x3<-rpois(20,1) df1<-data.frame(x1,x2,x3) df1
The following dataframe is created
x1 x2 x3 1 2 4 3 2 7 1 3 3 3 0 2 4 3 1 1 5 3 1 1 6 2 0 1 7 2 3 0 8 5 4 0 9 7 3 3 10 6 1 1 11 11 1 0 12 4 1 2 13 1 1 1 14 2 2 1 15 6 1 2 16 3 5 1 17 3 0 2 18 4 1 3 19 7 3 3 20 5 0 0
To extract values in x3 column that matches with values in V1 on the above created data frame, add the following code to the above snippet −
x1<-rpois(20,5) x2<-rpois(20,2) x3<-rpois(20,1) df1<-data.frame(x1,x2,x3) df1[df1$x3 %in% V1,"x3"] V1<-c(0,1)
Output
If you execute all the above given snippets as a single program, it generates the following Output −
[1] 1 1 1 0 0 1 0 1 1 1 0
Example 2
Following snippet creates a sample data frame and vector −
y1<-sample(1:100,20) y2<-sample(1:100,20) df2<-data.frame(y1,y2) df2
The following dataframe is created
y1 y2 1 3 93 2 37 64 3 73 84 4 43 46 5 51 91 6 99 7 7 38 71 8 78 82 9 93 73 10 20 8 11 63 58 12 1 50 13 60 40 14 46 33 15 58 97 16 41 3 17 44 77 18 68 51 19 42 75 20 94 11
To extract values in y2 column that matches with values in V2 on the above created data frame, add the following code to the above snippet −
y1<-sample(1:100,20) y2<-sample(1:100,20) df2<-data.frame(y1,y2) df2[df2$y2 %in% V2,"y2"] V2<-c(5,10,15,25,30,35,40,45,50,55,60,65,70,75,80,85,90,95)
Output
If you execute all the above given snippets as a single program, it generates the following Output −
[1] 50 40 75
Example 3
Following snippet creates a sample data frame and vector −
z1<-round(rnorm(20),0) z2<-round(rnorm(20),0) z3<-round(rnorm(20),0) df3<-data.frame(z1,z2,z3) df3
The following dataframe is created
z1 z2 z3 1 -1 0 0 2 0 -2 0 3 0 0 1 4 0 -1 -1 5 0 0 1 6 -1 -1 0 7 1 -1 0 8 0 0 -1 9 0 -1 -2 10 0 1 2 11 1 0 0 12 0 0 1 13 -1 0 -1 14 -1 -1 0 15 -3 0 0 16 -1 0 1 17 0 0 1 18 -1 1 -1 19 0 0 0 20 -1 1 -2
To extract values in z1 column that matches with values in V3 on the above created data frame, add the following code to the above snippet −
z1<-round(rnorm(20),0) z2<-round(rnorm(20),0) z3<-round(rnorm(20),0) df3<-data.frame(z1,z2,z3) df3[df3$z1 %in% V3,"z1"] V3<-c(0,1)
Output
If you execute all the above given snippets as a single program, it generates the following Output −
[1] 0 0 0 0 1 0 0 0 1 0 0 0