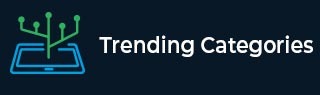
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Expressive words problem case in JavaScript
Sometimes people repeat letters to represent extra feeling, such as "hello" −> "heeellooo", "hi" −> "hiiii". In these strings like "heeellooo", we have groups of adjacent letters that are all the same: "h", "eee", "ll", "ooo".
For some given string S, a query word is stretchy if it can be made to be equal to S by any number of applications of the following extension operation: choose a group consisting of characters c, and add some number of characters c to the group so that the size of the group is 3 or more.
For example, starting with "hello", we could do an extension on the group "o" to get "hellooo", but we cannot get "helloo" since the group "oo" has size less than 3. Also, we could do another extension like "ll" −> "lllll" to get "helllllooo". If S = "helllllooo", then the query word "hello" would be stretchy because of these two extension operations: query = "hello" −> "hellooo" −> "helllllooo" = S.
Given a list of query words, we are required to return the number of words that are stretchy.
For example −
If the input string is −
const str = 'heeellooo';
And the list of words is −
const words = ["hello", "hi", "helo"];
And the output should be −
const output = 1
Example
The code for this will be −
const str = 'heeellooo'; const words = ["hello", "hi", "helo"]; const extraWords = (str, words) => { let count = 0; for (let w of words) { let i = 0; let j = 0; for (; i < str.length && j < w.length && w[j] === str[i];) { let lenS = 1; let lenW = 1; for (; i+lenS < str.length && str[i+lenS] === str[i]; lenS++); for (; j+lenW < w.length && w[j+lenW] === w[j]; lenW++); if (lenS < lenW || lenS > lenW && lenS < 3) break; i += lenS; j += lenW; } if (i === str.length && j === w.length) { count++; } } return count; } console.log(extraWords(str, words));
Output
And the output in the console will be −
1