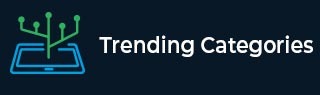
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Express.js – Getting Remote Client Address
An application needs to maintain the remote client address for managing the number of requests from a computer system and tracking its usage. But sometimes, the server runs behind an NGINX server, so we need to check its forward request. For this purpose, we can use "x-forwarded-for"
Syntax
req.headers['x-forwarded-for'] || req.socket.remoteAddress
If the proxy isn't yours, one should be careful while using the x-forwarded-for header, because it can be spoofed.
Example 1
Create a file with the name "remoteAddress.js" and copy the following code snippet. After creating the file, use the command "node remoteAddress.js" to run this code as shown in the example below −
// req.query() Demo Example // Importing the express module var express = require('express'); // Initializing the express and port number var app = express(); var PORT = 3000; // Getting the request query string app.get('/api', function(req, res){ console.log('id: ' + req.query.id) res.send('id: ' + req.query.id); }); // Listening to the port app.listen(PORT, function(err){ if (err) console.log(err); console.log("Server listening on PORT", PORT); });
Hit a Get Request at the following endpoint - http://localhost:3000/
Output
C:\home
ode>> node remoteAddress.js Server listening on PORT 3000 IP Address: ::ffff:127.0.0.1
Example 2
Let's take a look at one more example.
// express.raw() Demo Example // Importing the express module var express = require('express'); // Initializing the express and port number var app = express(); var PORT = 3000; app.get('/api*', function (req, res){ var ip = req.headers['x-forwarded-for'] || req.socket.remoteAddress console.log('IP Address: ' + ip) }); // Listening to the port app.listen(PORT, function(err){ if (err) console.log(err); console.log("Server listening on PORT", PORT); }); app.get('/', function (req, res){ var forwardedIpsStr = req.header('x-forwarded-for'); var IP = ''; if (forwardedIpsStr) { IP = forwardedIps = forwardedIpsStr.split(',')[0]; } });
Hit a Get Request for any of the following endpoints –
http://localhost:3000/api http://localhost:3000/api/v2 http://localhost:3000/api/v3 and so on...
It will compare all the endpoints with /api and track it.
Output
C:\home
ode>> node remoteAddress.js Server listening on PORT 3000 IP Address: ::ffff:127.0.0.1 IP Address: ::ffff:127.0.0.1 IP Address: ::ffff:127.0.0.1