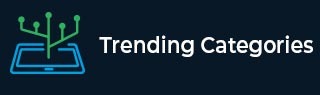
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Express.js – app.route() Method
The app.route() method returns the instance of a single route. This single route can be used to handle the HTTP verbs with the optional middleware. This method is mainly used to avoid duplicate names.
Syntax
app.route( )
Example 1
Create a file with the name "appRoute.js" and copy the following code snippet. After creating the file, use the command "node appRoute.js" to run this code.
// app.route() Demo Example // Importing the express module var express = require('express'); // Initializing the express and port number var app = express(); var PORT = 3000; // Creating a get, post & other requests app.route('/user') .get((req, res, next) => { res.send('GET is called'); console.log('GET is called'); }) .post((req, res, next) => { res.send('POST is called'); console.log('POST is called') }) .all((req, res, next) => { res.send('All others are called'); console.log('All others are called') }) app.listen(PORT, function(err){ if (err) console.log(err); console.log("Server listening on PORT", PORT); });
Hit the below endpoints/API to call the above function −
Output
C:\home
ode>> node appRoute.js '' '/api' '/api/v1'
Example 2
Let’s take a look at one more example.
// app.route() Demo Example // Importing the express module var express = require('express'); // Initializing the express and port number var app = express(); var PORT = 3000; // Creating a get, post & other requests app.route('/api/*') .get((req, res, next) => { res.send('GET is called'); console.log('GET is called'); }) .post((req, res, next) => { res.send('POST is called'); console.log('POST is called') }) .all((req, res, next) => { res.send('All others are called'); console.log('All others are called') }) app.listen(PORT, function(err){ if (err) console.log(err); console.log("Server listening on PORT", PORT); });
For the above function, you can call any endpoint after /api/ and it will be passed through the above function only. For example,
http://localhost:3000/api/v1,
.http://localhost:3000/api/user/path, etc
Output
C:\home
ode>> node appRoute.js Server listening on PORT 3000 All others are called GET is called
Advertisements