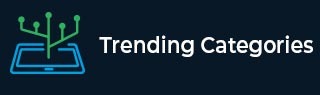
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Explain the purpose of the ‘in’ operator in JavaScript
This tutorial will teach about the ‘in’ operator in JavaScript. There are many operators available in JavaScript, such as arithmetic operators to perform mathematical operations, assignment operators, equality operators, etc. The ‘in’ operator is also one of them, which we can use to find properties from the object.
Before we start, let me ask you a question. Have you ever needed to check for the existence of the object's properties while coding with JavaScript? If yes, how did you deal with it? The answer is simple you can use the ‘in’ operator, which returns the Boolean value based on whether the object contains the property.
Use the ‘in’ operator to check the existence of object property
The ‘in’ operator works the same as other operators. It takes two operands. The object property as a left operand, and the object itself as a right operand.
Syntax
You can follow the syntax below to check for the existence of the object property using the ‘in’ operator.
let object = { property: value, } let ifExist = "property" in object;
In the above syntax, you can see how the object contains the property and its value. The value can be of type number, string, boolean, etc. The ifExist variable stores the true or false boolean value according to whether the property exists in the object.
Example 1
In this example, we have created the object containing the different properties and values. Also, the object contains the method. After that, we used the ‘in’ operator to check whether property exists in the object.
In the example output, users can observe that the ‘in’ operator returns true for property1 and property4 but false for property7 as it doesn’t exist in the object.
<html> <body> <h3>Using the <i> in operator </i> to check for the existence of the property in the object.</h3> <div id = "output"> </div> <script> let output = document.getElementById("output"); let object = { property1: "value", property2: 20, property3: false, property4: () => { console.log("This is a sample function."); }, }; let ifExist = "property1" in object; output.innerHTML += "The property1 exist in the object " + ifExist + "<br/>"; ifExist = "property4" in object; output.innerHTML += "The property4 exist in the object " + ifExist + "<br/>"; ifExist = "property7" in object; output.innerHTML += "The property7 exist in the object " + ifExist + "<br/>"; </script> </body> </html>
In JavaScript, every object has its prototype. The prototype chain object contains some methods and properties in the object virtually. However, we haven’t added those properties to the object, but JavaScript adds them by default. For example, the array and string prototype contain the ‘length’ property, and the object prototype contains the ‘toString’ property.
Example 2
In the example below, we have created the class and defined the constructor inside that to initialize the object properties. Also, we have defined the getSize() method inside the table class.
After that, we used the constructor function to create the object of the table class. We used the ‘in’ operator to check whether the property exists in the object prototype. In JavaScript, every object contains the toString() method in its prototype, that’s why it returns true.
<html> <body> <h3>Using the <i> in operator </i> to check for the existence of the property in the object prototype</h3> <div id = "output"> </div> <script> let output = document.getElementById("output"); // creating the table class class table { // constructor function constructor(prop1, prop2, prop3) { this.prop1 = prop1; this.prop2 = prop2; this.prop3 = prop3; } getSize() { return 10; } } // creating the object of the table class let tableObjet = new table("blue", "wood", "four drawers"); // check if a property exists in the object let ifExist = "prop1" in tableObjet; output.innerHTML += "The prop1 exists in the constructor properties of tableObject: " + ifExist + "</br>"; // some properties also exist in the object prototype chain. ifExist = "length" in tableObjet; output.innerHTML += "The length property exists in the constructor properties of tableObject: " + ifExist + "</br>"; ifExist = "toString" in tableObjet; output.innerHTML += "The toString Property exists in the constructor properties of tableObject: " + ifExist + "</br>"; </script> </body> </html>
Use the ‘in’ operator to check whether an index exists in the array
We can use the ‘in’ operator with objects only. The array is also an instance of the object. Users can use the instanceOf or typeOf operator to check the array type, which returns ‘Object’. So, keys in the array are array indexes, and values for the keys are array values.
Here, we can use the ‘in’ operator to check whether the index exists in the array. If it exists, we can access the array values to avoid the arrayOutOfBound exception.
Syntax
Users can follow the syntax below to check whether an index exists in the array −
let array = [10, 20, 30]; let ifExist = 2 in array;
In the above syntax, 2 written before in the operator is the array index but not the value.
Example 3
In the example below, we have created the array and checked the type of the array using the typeOf operator, which returns the ‘Object’.
Also, we have used the ‘in’ operator to check for the existence of the array index and length property in the array prototype.
<html> <body> <h2>Using the <i> in operator </i> to check whether the array index exists.</h2> <div id = "output"> </div> <script> let output = document.getElementById("output"); let array = [10, 20, "Hello", "Hi", true]; output.innerHTML += "The type of the array is " + typeof array + "<br/>"; let ifExist = 2 in array; output.innerHTML += "The index 2 exist in the array is " + ifExist + "<br/>"; ifExist = 7 in array; output.innerHTML += "The index 7 exist in the array is " + ifExist + "<br/>"; ifExist = "length" in array; output.innerHTML += "The length property exist in the array is " + ifExist + "<br/>"; </script> </body> </html>
This tutorial taught us to use the ‘in’ operator with objects and arrays. In the object, users can check for the existence of the property, and in the array, users can check for the existence of the index using the ‘in’ operator.