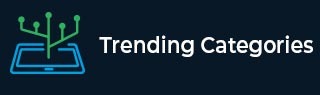
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Explain character classes in Java regular expressions
Java regular expressions, is often known as rege. It is employed for searching and manipulating text. They are utilized in numerous applications. Online scraping, email screening, and password validation are a few of them.A pattern that can be employed to match a specific character sequence in a string is also known as a regular expression. To build the pattern, a special syntax involving quantifiers, character classes, wildcard characters, and ordinary characters is employed.
Character classes are characters that can be matched by a regular expression. They are defined using square brackets []. For instance, the character class [abc] matches the characters a, b, and c. A range of characters can also be specified using hyphens. The character class [a-z] matches all lowercase letters.
Characters classes in regular expressions
Character class |
Description |
---|---|
[abc] |
It matches one character from characters "a," "b," or "c." |
[^\^abc] |
It matches a character that doesn't fit in the sets "a," "b," or "c." |
[a-c] |
It is compatible with multiple characters, starting from 'a' to 'c.' |
[a-c[f-h]] |
It is compatible with any character from the sets 'a-c' or 'f-h.' |
[a-c&&[b-c]] |
It is compatible with any character from 'a-c' and 'b-c' sets. |
[a-c&&[\^b-c]] |
It matches a character out of the 'a-c' set but not in the 'b-c' set. |
Example 1
This Java code explains how to match patterns using regular expressions. The code loads the necessary regex libraries and creates a class called RegexExample. Within the main function, the Pattern.matches method is employed to check if the strings match the pattern "[abc].ā It indicates they must contain either 'a', 'b', or 'c.'
Algorithm
Step 1: Import the regular expression library using import java.util.regex.*;.
Step 2: Define a class named RegexExample.
Step 3: Start the main method.
Step 4: Use Pattern.matches to check if a string matches a pattern.
Step 5: Test if the string "abc" matches the pattern "[abc]". Result: false.
Step 6: Test if the string "a" matches the pattern "[abc]". Result: true.
Step 7: Test if the string "abcd" matches the pattern "[abc]". Result: false.
Step 8: Print the results.
Example
import java.util.regex.*; public class RegexExample { public static void main(String args[]) { System.out.println(Pattern.matches("[abc]", "abc")); System.out.println(Pattern.matches("[abc]", "a")); System.out.println(Pattern.matches("[abc]", "abcd")); } }
Output
false true false
Example 2
The code employs the Java regular expression library to check if a string matches a certain pattern. The first pattern matches any lowercase letter from 'a' to 'f.ā The second pattern matches:
A letter from 'a' to 'zā
Uppercase letter from 'A' to 'Z'
or A digit from '0' to '9.ā
The third will be compatible with a lowercase/ uppercase letter that is not 'r', 's', or 't'. The output is true if the string matches the pattern otherwise it will be false.
Algorithm
Step 1: Employ Pattern.matches to check if a string matches a given pattern.
Step 2: Check if the string "d" matches the pattern "[a-f]". Result: true.
Step 3: Print the result of the first match. Output: true.
Step 4: Reuse Pattern.matches to check if the string "E" matches the pattern "[a-zA-G0-9]". Result: true.
Step 5: Print the result of the second match. Output: true.
Step 6: Reuse Pattern.matches to check if the string "r" matches the pattern "[a-zA-Z&&[^rst]]". Result: false.
Step 7: Print the result of the third match. Output: false.
Step 8: End of the main method.
Example
import java.util.regex. * ; public class RegexInstance { public static void main(String[] args) { boolean isMatch = Pattern.matches("[a-f]", "d"); System.out.println(isMatch); isMatch = Pattern.matches("[a-zA-G0-9]", "E"); System.out.println(isMatch); isMatch = Pattern.matches("[a-zA-Z&&[^rst]]", "r"); System.out.println(isMatch); } }
Output
true true false
Example 3
In the provided Java code, regular expressions are used for pattern matching. The program checks various strings against specific patterns, such as matching any character followed by 'g' or 'f'. The output confirms matches for "fg" and "mnv," while the other test cases don't match the patterns.
Algorithm
Step 1: Start the main method.
Step 2: Employ Pattern.matches to check if a string matches a specified pattern.
Step 3: Check if the string "fg" matches the pattern ".g". The dot . matches any single character, so ".g" matches any two-character string ending with "g". Result: true.
Step 4: Print the result of the first match. Output: true.
Step 5: Reuse Pattern.matches to check if the string "de" matches the pattern ".f". Here, ".f" matches any two-character string ending with "f". Result: false.
Step 6: Print the result of the second match. Output: false.
Step 7: Reuse Pattern.matches to check if the string "xyz" matches the pattern ".q". Here, ".q" matches any two-character string ending with "q". Result: false.
Step 8: Print the result of the third match. Output: false.
Step 9: Reuse Pattern.matches to check if the string "gce" matches the pattern ".c". Here, ".c" matches any two-character string ending with "c". Result: false.
Step 10: Print the result of the fourth match. Output: false.
Step 11: Reuse Pattern.matches to check if the string "mnv" matches the pattern "..v". Here, "..v" matches any three-character string ending with "v". Result: true.
Step 12: Print the result of the fifth match. Output: true.
Step 13: End the main method.
Example
import java.util.regex.Pattern; public class RegexInstance2 { public static void main(String args[]){ System.out.println(Pattern.matches(".g", "fg")); System.out.println(Pattern.matches(".f", "de")); System.out.println(Pattern.matches(".q", "xyz")); System.out.println(Pattern.matches(".c", "gce")); System.out.println(Pattern.matches("..v", "mnv")); } }
Output
true false false false true
Conclusion
In a nutshell, character classes in Java regular expressions play a significant role in text manipulation operations. They are employed to specify sets of characters that a regex pattern can match. These classes are denoted by square brackets [], and they allow you to match specific characters, ranges of characters, or even combinations of characters. Character classes allow you to design versatile and strong patterns that aid in activities like as password validation, email screening, and site scraping. These examples show how Java code can use character classes to recognize patterns inside strings and make smart decisions based on the matching results.