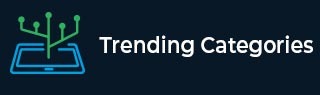
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Error.prototype.toString() Method in JavaScript
JavaScript is a widely-used programming language known for its flexibility and ease of use. One of the key features of the language is its ability to throw and handle errors. The Error.prototype.toString() method is a built-in method in JavaScript that allows developers to create and manipulate error objects. In this tutorial, we will discuss the basics of this method, including its syntax, usage, and some examples of how it can be used in real-world applications.
Error.prototype.toString() Method
The Error.prototype.toString() method is a built-in method in the JavaScript language that is used to convert an error object to a string. This method returns a string that contains the name of the error, followed by a colon (:), a space, and the error message. The error name is usually the name of the constructor function that was used to create the error object. For example, if you create an error object using the Error() constructor, the error name will be "Error".
Syntax
The syntax for the Error.prototype.toString() method is as follows −
errorObject.toString()
Where errorObject is an instance of an error object, such as an object created with the Error() constructor.
Usage
The Error.prototype.toString() method is used to convert an error object to a string. This can be useful when you need to output an error message to the console or display it on the screen.
In addition to its use for outputting error messages, the toString() method can also be used to perform other tasks, such as logging errors to a file or sending error messages to a remote server for further analysis.
Example 1
Simple Usage
Here are a few examples of how the Error.prototype.toString() method can be used in real-world applications −
<html> <body> <p id="print"></p> <script> try { throw new Error('An error occurred'); } catch (error) { document.getElementById("print").innerHTML = error.toString(); } </script> </body> </html>
In this example, we throw a new error using the Error() constructor and catch it using a try...catch block. Inside the catch block, we use the toString() method to convert the error object to a string and output it to the console.
Example 2
Custom Error Types
<html> <head> <title>Custom Error Types</title> </head> <body> <p id="print"></p> <script> class CustomError extends Error { constructor(message) { super(message); this.name = 'CustomError'; } } try { throw new CustomError('An error occurred'); } catch (error) { document.getElementById("print").innerHTML = error.toString(); // Output: "CustomError: An error occurred" } </script> </body> </html>
In this example, we define a custom error class called CustomError that inherits from the built-in Error class. The custom error class has a constructor that takes a message argument, which is passed to the super() method to set the error message. We also set the name property of the error object to "CustomError".
Next, we throw an instance of CustomError and catch it. Inside the catch block, we use the toString() method to convert the error object to a string and output it to the console. As you can see, the output contains the custom error name "CustomError" rather than the default "Error".
Example 3
Handling Errors in Asynchronous Code
<html> <head> <title>Handling Errors in Asynchronous Code</title> </head> <body> <p id="printpre"></p> <p id="print"></p> <script> async function fetchData() { try { const response = await fetch('https://example.com'); //put a GET link here if (!response.ok) { throw new Error(`HTTP error: ${response.status}`); } const data = await response.json(); document.getElementById("printpre").innerHTML=data; } catch (error) { console.log(error.toString()); document.getElementById("print").innerHTML=error.toString(); } } fetchData(); </script> </body> </html>
This example uses the fetch() API to make a request to a remote server and uses the try...catch block to handle any errors that occur during the request. Inside the try block, we check the ok property of the response object, if it is not ok, we throw an error with message "HTTP error: ${response.status}", which include HTTP status code of the response. If the request is successful, the fetchData() method will parse the json data and log it to the console. Finally, in the catch block, we use the toString() method to convert the error object to a string and output it to the console.
Conclusion
The Error.prototype.toString() method is a powerful and versatile tool for handling errors in JavaScript. It allows developers to easily convert error objects to strings, making it simple to output error messages to the console or other logging systems. Additionally, the use of custom error types with this method can make it more accurate and more readable for debugging. It's a great addition to any developer's tool belt and should be considered when working with error handling in JavaScript.