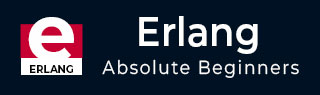
- Erlang Tutorial
- Erlang - Home
- Erlang - Overview
- Erlang - Environment
- Erlang - Basic Syntax
- Erlang - Shell
- Erlang - Data Types
- Erlang - Variables
- Erlang - Operators
- Erlang - Loops
- Erlang - Decision Making
- Erlang - Functions
- Erlang - Modules
- Erlang - Recursion
- Erlang - Numbers
- Erlang - Strings
- Erlang - Lists
- Erlang - File I/O
- Erlang - Atoms
- Erlang - Maps
- Erlang - Tuples
- Erlang - Records
- Erlang - Exceptions
- Erlang - Macros
- Erlang - Header Files
- Erlang - Preprocessors
- Erlang - Pattern Matching
- Erlang - Guards
- Erlang - BIFS
- Erlang - Binaries
- Erlang - Funs
- Erlang - Processes
- Erlang - Emails
- Erlang - Databases
- Erlang - Ports
- Erlang - Distributed Programming
- Erlang - OTP
- Erlang - Concurrency
- Erlang - Performance
- Erlang - Drivers
- Erlang - Web Programming
- Erlang Useful Resources
- Erlang - Quick Guide
- Erlang - Useful Resources
- Erlang - Discussion
Erlang - If statement
The first decision making statement we will look at is the ‘if’ statement. The general form of this statement in Erlang is shown in the following program −
Syntax
if condition -> statement#1; true -> statement #2 end.
In Erlang, the condition is an expression which evaluates to either true or false. If the condition is true, then statement#1 will be executed else statement#2 will be executed.
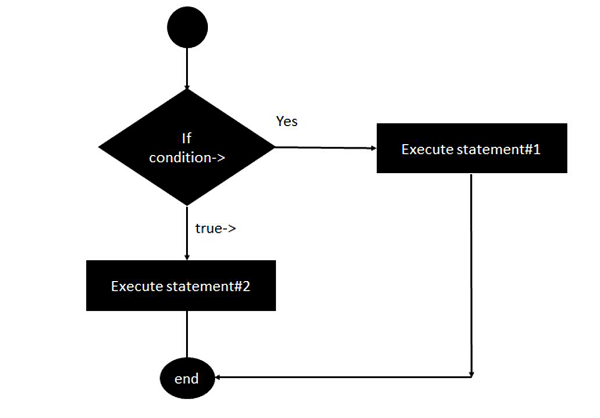
The following program is an example of the simple if expression in Erlang −
Example
-module(helloworld). -export([start/0]). start() -> A = 5, B = 6, if A == B -> io:fwrite("True"); true -> io:fwrite("False") end.
The following important things need to be noted about the above program −
The expression being used here is the comparison between the variables A and B.
The -> operator needs to follow the expression.
The ; needs to follow statement#1.
The -> operator needs to follow the true expression.
The statement ‘end’ needs to be there to signify the end of the ‘if’ block.
The output of the above program will be −
Output
False