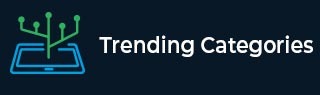
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Enums in Rust Programing
Also referred to as enumerations, enums are very useful in certain cases. In Rust, we use enums, as they allow us to define a type that may be one of a few different variants.
Enumerations are declared with the keyword enum.
Example
#![allow(unused)] #[derive(Debug)] enum Animal { Dog, Cat, } fn main() { let mut b : Animal = Animal::Dog; b = Animal::Cat; println!("{:?}",b); }
Output
Cat
Zero-variant Enums
Enums in Rust can also have zero variants, hence the name Zero-variant enums. Since they do not have any valid values, they cannot be instantiated.
Zero-variant enums are equivalent to the never type in Rust.
Example
#![allow(unused)] #[derive(Debug)] enum ZeroVariantEnum {} fn main() { let x: ZeroVariantEnum = panic!(); println!("{:?}",x); }
Output
thread 'main' panicked at 'explicit panic', src/main.rs:7:30
Advertisements