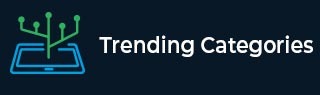
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Encode and decode MIME quoted-printable data using Python
Many times we need to deal with data which not always has the regular ASCII characters. For example, an email in a different language other than English. Python has mechanism to deal with such characters by using MIME (Multipurpose Internet Mail Extensions) based module. In this article we will see how we can decode such characters in an email or otherwise in some straight inputs.
Using email package
The email package has modules namely mime and charset which can carry out the encoding and decoding work as shown in the below example. We have taken an email message containing Unicode characters and then encoded it to utf-8.
Example
import email.mime, email.mime.nonmultipart, email.charset msg=email.mime.nonmultipart.MIMENonMultipart('text', 'plain', charset='utf-8') #Construct a new charset cs=email.charset.Charset('utf-8') cs.body_encoding = email.charset.QP # Set the content using the new charset msg.set_payload(u'This is the text containing ünicöde', charset=cs) print(msg)
Running the above code gives us the following result −
Output
Content-Type: text/plain; charset="utf-8" MIME-Version: 1.0 Content-Transfer-Encoding: quoted-printable This is the text containing =C3=BCnic=C3=B6de
Using quopri
This python module performs quoted-printable transport encoding and decoding. The quoted-printable encoding is designed for data where there are relatively few nonprintable characters. In the below example we see how we can encode and decode string with non regular ASCII characters.
Example
import quopri str1 = 'äé' #encoded = quopri.encodestring('äé'.encode('utf-8')) encoded = quopri.encodestring(str1.encode('utf-8')) print(encoded) str2 = '=C3=A4=C3=A9' decoded_string = quopri.decodestring(str2) print(decoded_string.decode('utf-8'))
Running the above code gives us the following result −
Output
b'=C3=A4=C3=A9' äé