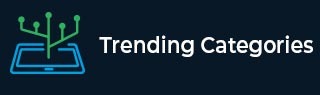
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Element equal to the sum of all the remaining elements in C++
In this problem, we are given an array arr[] consisting of n positive values. Our task is to find the element equal to the sum of all the remaining elements of the array.
Code Description: We need to find the element whose value is equal to the sum of all elements of the array except that element.
Let’s take an example to understand the problem,
Input: arr[] = { 5, 4, 17, 1, 7 }
Output: 17
Explanation −
The sum of the rest of the element is (5 + 4 + 1 + 7 ) = 17, which is equal to the remaining element 17.
Solution Approach −
A simple solution to the problem is using the fact that the sum of all elements of the array is twice the given element. For this we will follow these steps,
Step 1: Find the sum of all elements of the array.
Step 2: loop for each element of the array,
Step 2.1: if arr[i] == sum/2.
Step 2.1.1: if true. Flag = 1, break the loop.
Step 2.2.1: if false, leave it.
Step 3: If flag == 1, print arr[i]
Step 4: Else, print “No such element found”.
Program to illustrate the working of our solution,
Example
#include <iostream> using namespace std; void findElemenetInArray(int arr[], int n) { int arraySum = 0; int flag = 0, i; for (i = 0; i < n; i++) arraySum += arr[i]; for (i = 0; i < n; i++) if ( (2*arr[i]) == arraySum ) { flag = 1; break; } if(flag) cout<<arr[i]; else cout<<"No such element is found!"; } int main() { int n = 5; int arr[n] = { 5, 4, 7, 1, 17 }; findElemenetInArray(arr, n); return 0; }
Output
17