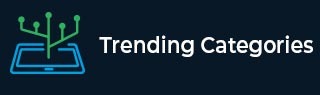
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Distribute Coins in Binary Tree in C++
Suppose we have the root of a binary tree with N nodes, here each node in the tree has node.val number of coins, and there are N coins in total. In one move, we can choose two adjacent nodes and move only one coin from one node to the another node. (The move may be from parent to child node, or from child to parent node.). We have to find the number of moves required to make every node have exactly one coin.
So if the tree is like −
Then the output will be 3. From the left child, send 2 coins to the root (one move for each coin, so total 2 moves), then move one coin from root to the right child, so in total there are 3 moves.
To solve this, we will follow these steps −
Define one recursive method called solve(), this will take a node called root
if root is null, then return 0
l := solve(left of the root)
r := solve(right of the root)
ans := |l| + |r|
return l + r + value of root – 1
in the main section, set ans := 0, call solve(root), then return ans
Example
#include <bits/stdc++.h> using namespace std; class TreeNode{ public: int val; TreeNode *left, *right; TreeNode(int data){ val = data; left = NULL; right = NULL; } }; void insert(TreeNode **root, int val){ queue<TreeNode*> q; q.push(*root); while(q.size()){ TreeNode *temp = q.front(); q.pop(); if(!temp->left){ if(val != NULL) temp->left = new TreeNode(val); else temp->left = new TreeNode(0); return; }else{ q.push(temp->left); } if(!temp->right){ if(val != NULL) temp->right = new TreeNode(val); else temp->right = new TreeNode(0); return; }else{ q.push(temp->right); } } } TreeNode *make_tree(vector<int> v){ TreeNode *root = new TreeNode(v[0]); for(int i = 1; i<v.size(); i++){ insert(&root, v[i]); } return root; } class Solution { public: int ans; int solve(TreeNode* root){ if(!root)return 0; int l = solve(root->left); int r = solve(root->right); ans += abs(l) + abs(r); return l + r + root->val - 1; } int distributeCoins(TreeNode* root) { ans = 0; solve(root); return ans; } }; main(){ vector<int> v = {0,3,0}; TreeNode *root = make_tree(v); Solution ob; cout << (ob.distributeCoins(root)); }
Input
[0,3,0]
Output
3